问题1:给定两个字符串str1和str2,输出两个字符串的最长公共子串,保证str1和str2的最长公共子串存在且唯一。 要求: (1)数据输入:字符串长度0<|str1|,|str2|≦5000,元素从{0-9,A-Z}随机生成。 (2)数据输出:最长公共子串。写出c++代码
时间: 2024-11-25 10:18:23 浏览: 29
要解决这个问题,可以使用动态规划的方法来找到两个字符串的最长公共子串。这里是一个C++实现的示例代码:
```cpp
#include <string>
#include <vector>
std::string longestCommonSubstring(const std::string& str1, const std::string& str2) {
int m = str1.length();
int n = str2.length();
std::vector<std::vector<int>> dp(m + 1, std::vector<int>(n + 1, 0));
int maxLength = 0;
int endIndex = -1;
for (int i = 1; i <= m; ++i) {
for (int j = 1; j <= n; ++j) {
if (str1[i - 1] == str2[j - 1]) {
dp[i][j] = dp[i - 1][j - 1] + 1;
if (dp[i][j] > maxLength) {
maxLength = dp[i][j];
endIndex = i - 1;
}
} else {
dp[i][j] = 0;
}
}
}
return str1.substr(endIndex - maxLength + 1, maxLength);
}
// 示例
int main() {
std::string str1 = "example123";
std::string str2 = "exampleregular";
std::string result = longestCommonSubstring(str1, str2);
std::cout << "Longest common substring: " << result << std::endl;
return 0;
}
```
这段代码定义了一个`longestCommonSubstring`函数,它通过遍历两个字符串,比较字符并更新动态规划矩阵`dp`来找出最长公共子串。如果找到更长的子串,就更新`maxLength`和`endIndex`。最后返回以`endIndex`为起始点,长度为`maxLength`的子串作为结果。
注意,这个代码假设输入字符串只包含大写字母和数字,如果实际输入可能包含其他字符,你需要相应地修改比较逻辑。
阅读全文
相关推荐

















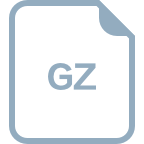