怎样java写出一个打飞机的游戏
时间: 2023-10-18 19:37:46 浏览: 35
打飞机游戏是一个经典的小游戏,使用 Java 可以实现。下面是一个简单的 Java 实现打飞机游戏的示例代码,供您参考:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class PlaneGame extends JPanel implements Runnable {
private static final long serialVersionUID = 1L;
private int x = 225, y = 600, width = 50, height = 50;
private int bg_y1 = -700, bg_y2 = -1500, bg_speed = 2;
private List<Bullet> bullets = new ArrayList<Bullet>();
private List<EnemyPlane> planes = new ArrayList<EnemyPlane>();
private boolean isAlive = true;
private int score = 0;
public PlaneGame() {
this.addKeyListener(new KeyAdapter() {
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT) {
x -= 10;
} else if (key == KeyEvent.VK_RIGHT) {
x += 10;
} else if (key == KeyEvent.VK_SPACE) {
bullets.add(new Bullet(x + width / 2, y - 10));
}
}
});
}
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.BLUE);
g.fillRect(x, y, width, height);
g.setColor(Color.WHITE);
g.drawString("分数:" + score, 20, 20);
g.setColor(Color.RED);
for (Bullet bullet : bullets) {
g.fillRect(bullet.getX(), bullet.getY(), bullet.getWidth(), bullet.getHeight());
}
g.setColor(Color.GREEN);
for (EnemyPlane plane : planes) {
g.fillRect(plane.getX(), plane.getY(), plane.getWidth(), plane.getHeight());
}
g.setColor(Color.BLACK);
g.fillRect(0, bg_y1, 500, 800);
g.fillRect(0, bg_y2, 500, 800);
}
public void run() {
while (isAlive) {
try {
Thread.sleep(20);
} catch (InterruptedException e) {
e.printStackTrace();
}
bg_y1 += bg_speed;
bg_y2 += bg_speed;
if (bg_y1 >= 800) {
bg_y1 = -1500;
}
if (bg_y2 >= 800) {
bg_y2 = -1500;
}
for (Bullet bullet : bullets) {
bullet.move();
if (bullet.getY() < 0) {
bullets.remove(bullet);
} else {
for (EnemyPlane plane : planes) {
if (bullet.getX() > plane.getX() && bullet.getX() < plane.getX() + plane.getWidth()
&& bullet.getY() > plane.getY() && bullet.getY() < plane.getY() + plane.getHeight()) {
bullets.remove(bullet);
planes.remove(plane);
score += 10;
}
}
}
}
for (EnemyPlane plane : planes) {
plane.move();
if (plane.getY() > 800) {
planes.remove(plane);
}
}
if (Math.random() < 0.01) {
planes.add(new EnemyPlane((int) (Math.random() * 450), -50));
}
for (EnemyPlane plane : planes) {
if (plane.getX() > x - plane.getWidth() && plane.getX() < x + width
&& plane.getY() > y - plane.getHeight() && plane.getY() < y + height) {
isAlive = false;
}
}
repaint();
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("打飞机");
PlaneGame game = new PlaneGame();
frame.add(game);
frame.setSize(500, 800);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
new Thread(game).start();
}
}
class Bullet {
private int x, y, width = 10, height = 10, speed = 10;
public Bullet(int x, int y) {
this.x = x;
this.y = y;
}
public void move() {
y -= speed;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
}
class EnemyPlane {
private int x, y, width = 50, height = 50, speed = 3;
public EnemyPlane(int x, int y) {
this.x = x;
this.y = y;
}
public void move() {
y += speed;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getWidth() {
return width;
}
public int getHeight() {
return height;
}
}
```
该代码使用了 Java 的面向对象编程思想,主要分为三个类:`PlaneGame`、`Bullet` 和 `EnemyPlane`。其中 `PlaneGame` 类是主类,继承于 `JPanel` 并实现了 `Runnable` 接口,用于显示游戏界面和控制游戏逻辑。`Bullet` 和 `EnemyPlane` 类分别表示子弹和敌机,用于维护游戏中的子弹和敌机列表以及移动逻辑。
在 `PlaneGame` 类的构造方法中,添加了键盘监听器,用于监听左、右方向键和空格键的按下事件,控制飞机的移动和发射子弹。
在 `PlaneGame` 类的 `paint` 方法中,使用 `Graphics` 对象绘制游戏界面。使用 `List` 维护了子弹和敌机列表,并在 `run` 方法中控制它们的移动、碰撞检测和删除。
在 `PlaneGame` 类的 `main` 方法中,创建了一个 `JFrame` 对象,将 `PlaneGame` 对象添加到其中,并启动一个新的线程来运行游戏。
该示例代码只是一个简单的打飞机游戏实现,您可以根据需要进行修改和扩展。
相关推荐
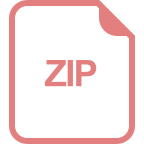
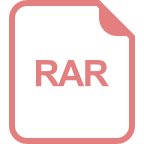
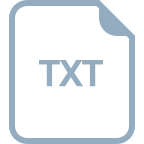














