用Java语言实现ftpclient删除文件目录
时间: 2024-03-06 10:51:00 浏览: 169
使用Java语言实现FTPClient删除远程服务器上的文件或目录,可以按照以下步骤进行操作:
1. 创建FTPClient对象,并连接到FTP服务器。
2. 使用FTPClient的login()方法登录FTP服务器。
3. 使用FTPClient的changeWorkingDirectory()方法切换到要删除的目录。
4. 使用FTPClient的deleteFile()方法删除指定的文件。
5. 使用FTPClient的removeDirectory()方法删除指定的目录。
6. 关闭FTP连接。
以下是Java代码示例:
```
import org.apache.commons.net.ftp.FTPClient;
import java.io.IOException;
public class FTPDeleteExample {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String pass = "password";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, pass);
String directoryPath = "/example/directory";
if (ftpClient.changeWorkingDirectory(directoryPath)) {
String fileName = "example.txt";
boolean deleted = ftpClient.deleteFile(fileName);
if (deleted) {
System.out.println("File " + fileName + " deleted successfully.");
} else {
System.out.println("Failed to delete file " + fileName + ".");
}
} else {
System.out.println("Directory " + directoryPath + " not found.");
}
String emptyDirectoryPath = "/empty/directory";
if (ftpClient.changeWorkingDirectory(emptyDirectoryPath)) {
boolean removed = ftpClient.removeDirectory(emptyDirectoryPath);
if (removed) {
System.out.println("Directory " + emptyDirectoryPath + " removed successfully.");
} else {
System.out.println("Failed to remove directory " + emptyDirectoryPath + ".");
}
} else {
System.out.println("Directory " + emptyDirectoryPath + " not found.");
}
ftpClient.logout();
} catch (IOException ex) {
System.err.println("Error: " + ex.getMessage());
} finally {
try {
ftpClient.disconnect();
} catch (IOException ex) {
System.err.println("Error: " + ex.getMessage());
}
}
}
}
```
在上面的代码中,使用FTPClient连接到FTP服务器,然后使用changeWorkingDirectory()方法切换到要删除的目录,并使用deleteFile()方法删除指定的文件,或使用removeDirectory()方法删除指定的目录。最后,关闭FTP连接。
阅读全文
相关推荐
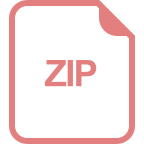
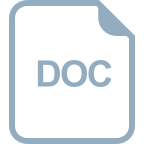
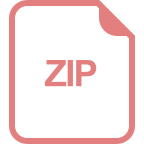
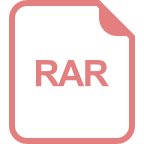
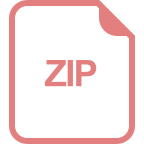
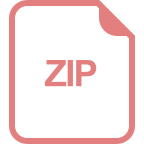
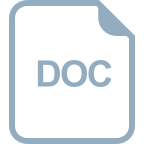

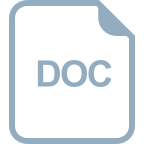
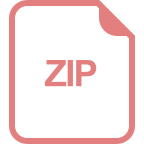
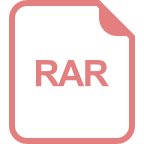
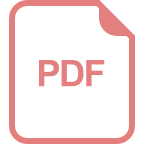
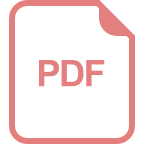
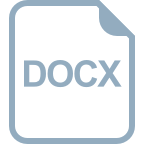
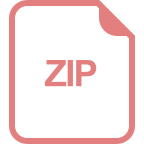
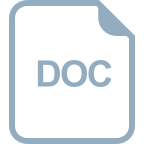