pytorch lstm
时间: 2023-07-23 10:12:00 浏览: 51
LSTM Attention是一种基于LSTM模型和注意力机制的深度学习模型,用于处理序列数据。在PyTorch中,可以使用torch.nn模块中的LSTM和Attention层来实现LSTM Attention模型。LSTM层用于处理序列数据的长期依赖关系,而Attention层则用于对序列中的不同部分进行加权,以便更好地捕捉序列中的重要信息。LSTM Attention模型在自然语言处理、语音识别等领域中得到了广泛应用。
相关问题
pytorch LSTM
PyTorch LSTM is an implementation of the Long Short-Term Memory (LSTM) neural network model using the PyTorch deep learning framework. LSTM models are a type of recurrent neural network (RNN) that are designed to model sequential data by capturing long-term dependencies and addressing the vanishing gradient problem that is common in traditional RNNs.
PyTorch LSTM models consist of multiple LSTM cells that are connected in a chain-like structure. Each LSTM cell has three gates - input, forget, and output - that control the flow of information through the cell. The input gate determines how much new information is added to the cell state, the forget gate decides how much old information is discarded from the cell state, and the output gate regulates the amount of information that is passed on to the next cell in the chain.
PyTorch provides a simple and intuitive API for building LSTM models, with pre-built modules and functions for constructing the various components of the model. The PyTorch LSTM module provides an easy way to construct a multi-layer LSTM model, with options for bidirectional processing and dropout regularization.
Overall, PyTorch LSTM is a powerful and flexible tool for modeling sequential data and has been used in a wide range of applications, including natural language processing, speech recognition, and time series prediction.
Pytorch lstm
PyTorch是一个开源的机器学习框,它提供了丰富的工具和库来构建和训练深度学习模型。LSTM(Long Short-Term Memory)是一种循环神经网络(RNN)的变体,它在处理序列数据时具有很强的表达能力。
在PyTorch中,可以使用torch.nn模块来构建LSTM模型。首先,需要导入相关的库和模块:
```python
import torch
import torch.nn as nn
```
然后,可以定义一个LSTM模型类,继承自nn.Module,并实现其中的forward方法:
```python
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(x.device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
```
在上述代码中,LSTMModel类接受输入大小(input_size)、隐藏状态大小(hidden_size)、LSTM层数(num_layers)和输出大小(output_size)作为参数。在forward方法中,首先初始化LSTM的隐藏状态和细胞状态,然后将输入数据传入LSTM模型,最后通过全连接层进行输出。
接下来,可以实例化LSTMModel类,并定义损失函数和优化器:
```python
input_size = 10
hidden_size = 20
num_layers = 2
output_size = 1
model = LSTMModel(input_size, hidden_size, num_layers, output_size)
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
```
最后,可以使用训练数据对模型进行训练:
```python
# 假设有训练数据 x 和目标值 y
# ...
# 将数据转换为张量
x = torch.tensor(x, dtype=torch.float32)
y = torch.tensor(y, dtype=torch.float32)
# 前向传播和计算损失
output = model(x)
loss = criterion(output, y)
# 反向传播和优化
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
这是一个简单的PyTorch LSTM模型的介绍和示例。你可以根据自己的需求进行模型的调整和训练。
相关推荐
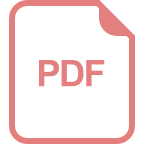












