pytorch lstm拟合
时间: 2024-06-13 18:03:06 浏览: 105
PyTorch中的LSTM(Long Short-Term Memory)是一种用于处理序列数据的强大工具,它在深度学习中特别适合于自然语言处理和时间序列预测任务。LSTM是一种循环神经网络(RNN)的一种变体,它解决了传统RNN中梯度消失或梯度爆炸的问题,使得模型能更好地捕捉长期依赖。
LSTM包含三个门控机制:输入门、遗忘门和输出门,它们控制着信息的流入、流出以及细胞状态的更新,允许模型在训练过程中选择性地保留或遗忘历史信息。下面是使用PyTorch创建一个基本LSTM模型并进行拟合的一般步骤:
1. 导入所需库:
```python
import torch
import torch.nn as nn
import torch.optim as optim
```
2. 定义LSTM层:
```python
class LSTMModel(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTMModel, self).__init__()
self.hidden_size = hidden_size
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
# 初始化隐藏状态和细胞状态
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
out, _ = self.lstm(x, (h0, c0)) # 返回的是output和最后一个hidden state
out = self.fc(out[:, -1, :]) # 取出最后一个时间步的输出
return out
```
3. 初始化模型、损失函数和优化器:
```python
input_size = ... # 输入数据的维度
hidden_size = ...
num_layers = ...
output_size = ... # 输出维度
model = LSTMModel(input_size, hidden_size, num_layers, output_size)
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
```
4. 准备数据和训练循环:
```python
# 假设 X_train和y_train是你的训练数据
X_train, y_train = ..., ...
inputs = torch.tensor(X_train).float().to(device)
labels = torch.tensor(y_train).long().to(device)
for epoch in range(num_epochs):
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
阅读全文
相关推荐
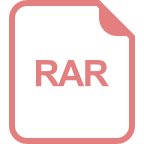
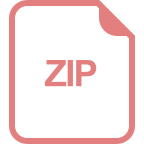
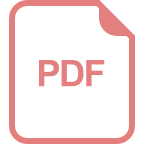















