修改#include <fstream> #include <iostream> using namespace std; struct Student { char name[100]; int* scores; double avg; }; Student* Input(char* file, int& stuNum, int& hwNum) { ifstream in(file, ios::in); int itemNum; in >> stuNum >> hwNum >> itemNum; // 定义和初始化学生列表 Student* stuList = new Student[stuNum]; for (int i = 0; i < stuNum; i++) { stuList[i].scores = new int[hwNum]; for (int j = 0; j < hwNum; j++) stuList[i].scores[j] = 0; } // 读入数据 int k = 0; for (int i = 0; i < itemNum; i++) { char name[100]; int time, score; in >> name >> time >> score; int j; for ( j = 0; j < k; j++) { if (strcmp(name, stuList[j].name) == 0) break; } if (j < k) { stuList[j].scores[time - 1] = score; } else { strcpy(stuList[k].name, name); stuList[k].scores[time - 1] = score; k++; } } in.close(); return stuList; } int main() { int stuNum, hwNum; Student* stuList = Input("score.txt", stuNum, hwNum); for (int i = 0; i < stuNum; i++) { cout << stuList[i].name << "\t"; for (int j = 0; j < hwNum; j++) cout << stuList[i].scores[j] << "\t"; cout << endl; } return 0; }使之能运行
时间: 2024-03-03 10:52:00 浏览: 118
这段代码看起来是读取一个文件 "score.txt",然后将文件中的数据存储到一个 Student 结构体数组中,并输出每个学生的姓名和成绩。但是,代码中有些问题,例如在结构体 Student 中没有定义析构函数,导致内存泄漏;并且没有进行输入文件是否存在的判断等等。
以下是修改后的代码:
```
#include <fstream>
#include <iostream>
#include <cstring>
using namespace std;
struct Student {
char name[100];
int* scores;
double avg;
~Student() { delete[] scores; } // 添加析构函数
};
Student* Input(char* file, int& stuNum, int& hwNum) {
ifstream in(file, ios::in);
if (!in) { // 判断文件是否打开成功
cerr << "Error: Cannot open file!" << endl;
exit(1);
}
int itemNum;
in >> stuNum >> hwNum >> itemNum;
// 定义和初始化学生列表
Student* stuList = new Student[stuNum];
for (int i = 0; i < stuNum; i++) {
stuList[i].scores = new int[hwNum];
for (int j = 0; j < hwNum; j++)
stuList[i].scores[j] = 0;
}
// 读入数据
int k = 0;
for (int i = 0; i < itemNum; i++) {
char name[100];
int time, score;
in >> name >> time >> score;
int j;
for (j = 0; j < k; j++) {
if (strcmp(name, stuList[j].name) == 0)
break;
}
if (j < k) {
stuList[j].scores[time - 1] = score;
}
else {
strcpy(stuList[k].name, name);
stuList[k].scores[time - 1] = score;
k++;
}
}
in.close();
return stuList;
}
int main() {
int stuNum, hwNum;
Student* stuList = Input("score.txt", stuNum, hwNum);
for (int i = 0; i < stuNum; i++) {
cout << stuList[i].name << "\t";
for (int j = 0; j < hwNum; j++)
cout << stuList[i].scores[j] << "\t";
cout << endl;
}
delete[] stuList; // 释放内存
return 0;
}
```
修改后的代码中,添加了析构函数来避免内存泄漏,同时在文件打开失败时进行了错误处理。最后在 main 函数中添加了释放内存的操作,以避免内存泄漏。
阅读全文
相关推荐
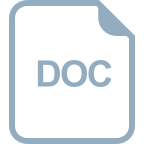
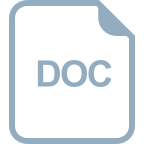
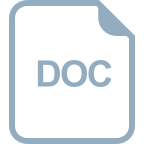











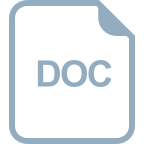
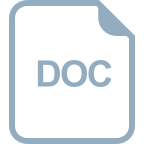
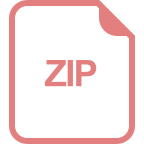