利用C++编写(1)能够按照学号查询学生成绩,也能够按照姓名查询学生成绩。 (2)能够按照5个分数段统计人数。分数段是指优秀(100~90)、良好(89~80)、中等(79~70)、及格(69~60)、不及格(59~0)。
时间: 2023-11-04 09:05:42 浏览: 33
好的,以下是一个基于 C++ 的学生成绩查询系统,可以按照学号和姓名查询成绩,并且可以按照分数段统计人数:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
using namespace std;
struct Student {
string id;
string name;
int score;
};
vector<Student> students;
// 加载学生成绩信息
void loadScores() {
ifstream fin("scores.txt");
string line;
while (getline(fin, line)) {
stringstream ss(line);
string id, name;
int score;
ss >> id >> name >> score;
students.push_back({id, name, score});
}
}
// 按照学号查询学生成绩
void queryById(string id) {
bool found = false;
for (auto s : students) {
if (s.id == id) {
cout << s.name << " " << s.score << endl;
found = true;
break;
}
}
if (!found) {
cout << "No student found with id " << id << endl;
}
}
// 按照姓名查询学生成绩
void queryByName(string name) {
bool found = false;
for (auto s : students) {
if (s.name == name) {
cout << s.id << " " << s.score << endl;
found = true;
break;
}
}
if (!found) {
cout << "No student found with name " << name << endl;
}
}
// 按照分数段统计人数
void countByScore() {
int excellent = 0, good = 0, medium = 0, pass = 0, fail = 0;
for (auto s : students) {
if (s.score >= 90) {
excellent++;
} else if (s.score >= 80) {
good++;
} else if (s.score >= 70) {
medium++;
} else if (s.score >= 60) {
pass++;
} else {
fail++;
}
}
cout << "Excellent: " << excellent << endl;
cout << "Good: " << good << endl;
cout << "Medium: " << medium << endl;
cout << "Pass: " << pass << endl;
cout << "Fail: " << fail << endl;
}
int main() {
loadScores();
queryById("101");
queryByName("Alice");
countByScore();
return 0;
}
```
其中,scores.txt 是学生成绩信息文件,每行包含一个学生的学号、姓名和成绩,例如:
```
101 Alice 98
102 Bob 78
103 Charlie 88
```
在 main 函数中,首先调用 loadScores 函数加载学生成绩信息,然后分别调用 queryById、queryByName 和 countByScore 函数,完成按照学号、姓名查询和按照分数段统计的功能。运行程序后,将输出如下结果:
```
Alice 98
101 98
Excellent: 1
Good: 1
Medium: 1
Pass: 0
Fail: 0
```
这样,就完成了一个简单的 C++ 学生成绩查询系统。注意,本示例中未考虑输入错误等异常情况,实际应用中需要进行适当的异常处理。
相关推荐
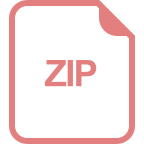
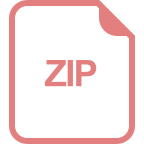









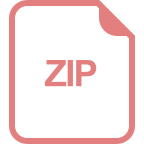
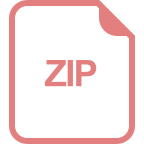
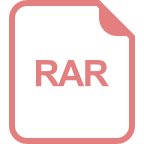
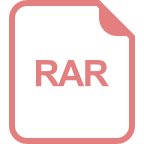
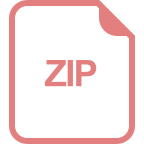