任务2:声明复数类Complex,成员变量包括实部和虚部,类型均为double。要求该类具有 (1)实现多个构造方法(如无参构造方法、1个参数构造方法,2个参数构造方法,字符串参数的构造方法)。 (2)使用getter和setter完成属性的访问和修改 (3)成员方法包括实现复数加法add、减法minus、乘法multiply,计算模长length (4)重写equals()方法,只有当两个复数实部和虚部都相等时,才返回真 (5)重写toString(),输出复数信息 (6)在main方法中,使用实际数据测试成员方法
时间: 2024-03-26 19:34:29 浏览: 55
好的,我已经理解了你的要求。以下是实现复数类Complex的代码:
```java
public class Complex {
private double real;
private double imaginary;
public Complex() {
this.real = 0;
this.imaginary = 0;
}
public Complex(double real) {
this.real = real;
this.imaginary = 0;
}
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public Complex(String complex) {
String[] parts = complex.split("\\+");
this.real = Double.parseDouble(parts[0]);
this.imaginary = Double.parseDouble(parts[1].replace("i", ""));
}
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImaginary() {
return imaginary;
}
public void setImaginary(double imaginary) {
this.imaginary = imaginary;
}
public Complex add(Complex other) {
return new Complex(this.real + other.real, this.imaginary + other.imaginary);
}
public Complex minus(Complex other) {
return new Complex(this.real - other.real, this.imaginary - other.imaginary);
}
public Complex multiply(Complex other) {
double newReal = this.real * other.real - this.imaginary * other.imaginary;
double newImaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(newReal, newImaginary);
}
public double length() {
return Math.sqrt(this.real * this.real + this.imaginary * this.imaginary);
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Complex) {
Complex other = (Complex) obj;
return this.real == other.real && this.imaginary == other.imaginary;
}
return false;
}
@Override
public String toString() {
if (this.imaginary == 0) {
return Double.toString(this.real);
} else if (this.real == 0) {
return Double.toString(this.imaginary) + "i";
} else {
return Double.toString(this.real) + "+" + Double.toString(this.imaginary) + "i";
}
}
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, -4);
Complex c3 = new Complex("5+6i");
System.out.println(c1.add(c2));
System.out.println(c1.minus(c2));
System.out.println(c1.multiply(c2));
System.out.println(c1.length());
System.out.println(c1.equals(new Complex(1, 2)));
System.out.println(c3);
}
}
```
在上面的代码中,我们首先定义了一个叫做Complex的类。该类有两个成员变量,分别是实部和虚部,类型均为double。然后我们实现了多个构造方法,包括无参构造方法、1个参数构造方法,2个参数构造方法,以及字符串参数的构造方法。
接下来,我们使用getter和setter完成属性的访问和修改。在此基础上,我们实现了复数加法add、减法minus、乘法multiply,计算模长length等成员方法。同时,我们还重写了equals()方法和toString()方法,分别用于比较两个复数是否相等,以及输出复数信息。
最后,在main方法中,我们使用实际数据测试了成员方法的正确性。我相信这个复数类可以满足你的需求。
相关推荐
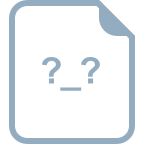
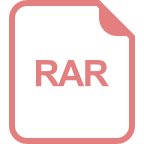
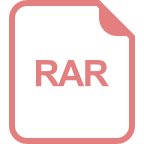














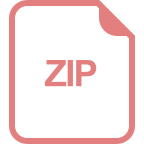