1)类的封装性 3-34,声明复数类Complex,成员变量包括实部和虚部,成员方法包括实现由字符串构造复数、复数加法、减法、字符串描述、比较相等、计算复数的模√实部+虚部等操作。复数语法图如图3-16所示。
时间: 2023-05-22 12:01:17 浏览: 169
答:可以使用以下代码实现:
```
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex(const std::string& str) {
std::stringstream ss(str);
char ch;
ss >> real >> ch >> imag;
}
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imag + other.imag);
}
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imag - other.imag);
}
bool operator==(const Complex& other) const {
return real == other.real && imag == other.imag;
}
double mod() const {
return std::sqrt(real * real + imag * imag);
}
std::string to_string() const {
std::stringstream ss;
ss << real << "+" << imag << "i";
return ss.str();
}
};
```
这个类实现了复数的加法、减法、比较相等、计算模以及字符串描述等操作。可以使用以下代码测试:
```
Complex c1(1, 2);
Complex c2("3+4i");
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
std::cout << c1.to_string() << std::endl;
std::cout << c2.to_string() << std::endl;
std::cout << c3.to_string() << std::endl;
std::cout << c4.to_string() << std::endl;
std::cout << (c1 == c2) << std::endl;
std::cout << c1.mod() << std::endl;
```
阅读全文
相关推荐
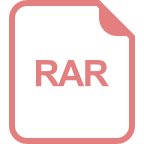
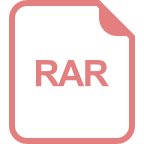
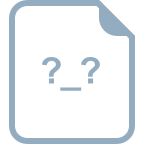














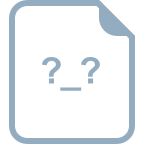
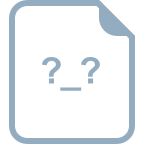