声明复数类Complex,成员变量包括实部和虚部,类型均为double。要求该类具有 (1)实现多个构造方法(如无参构造方法、1个参数构造方法,2个参数构造方法,字符串参数的构造方法)。 (2)使用getter和setter完成属性的访问和修改 (3)成员方法包括实现复数加法add、减法minus、乘法multiply,计算模长length (4)重写equals()方法,只有当两个复数实部和虚部都相等时,才返回真 (5)重写toString(),输出复数信息 (6)在main方法中,使用实际数据测试成员方法
时间: 2024-02-12 21:08:17 浏览: 17
下面是实现上述要求的复数类 Complex:
```java
public class Complex {
private double real;
private double imaginary;
public Complex() {
this.real = 0.0;
this.imaginary = 0.0;
}
public Complex(double real) {
this.real = real;
this.imaginary = 0.0;
}
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
public Complex(String complexString) {
String[] parts = complexString.split("\\+|i");
this.real = Double.parseDouble(parts[0]);
this.imaginary = Double.parseDouble(parts[1]);
}
public double getReal() {
return this.real;
}
public double getImaginary() {
return this.imaginary;
}
public void setReal(double real) {
this.real = real;
}
public void setImaginary(double imaginary) {
this.imaginary = imaginary;
}
public Complex add(Complex other) {
double real = this.real + other.real;
double imaginary = this.imaginary + other.imaginary;
return new Complex(real, imaginary);
}
public Complex minus(Complex other) {
double real = this.real - other.real;
double imaginary = this.imaginary - other.imaginary;
return new Complex(real, imaginary);
}
public Complex multiply(Complex other) {
double real = this.real * other.real - this.imaginary * other.imaginary;
double imaginary = this.real * other.imaginary + this.imaginary * other.real;
return new Complex(real, imaginary);
}
public double length() {
return Math.sqrt(this.real * this.real + this.imaginary * this.imaginary);
}
@Override
public boolean equals(Object other) {
if (this == other) {
return true;
}
if (!(other instanceof Complex)) {
return false;
}
Complex otherComplex = (Complex) other;
return this.real == otherComplex.real && this.imaginary == otherComplex.imaginary;
}
@Override
public String toString() {
if (this.imaginary >= 0) {
return String.format("%.2f+%.2fi", this.real, this.imaginary);
} else {
return String.format("%.2f%.2fi", this.real, this.imaginary);
}
}
public static void main(String[] args) {
Complex c1 = new Complex(1.0, 2.0);
Complex c2 = new Complex(3.0, 4.0);
Complex c3 = new Complex("5+6i");
System.out.println("c1 = " + c1.toString());
System.out.println("c2 = " + c2.toString());
System.out.println("c3 = " + c3.toString());
Complex c4 = c1.add(c2);
System.out.println("c1 + c2 = " + c4.toString());
Complex c5 = c1.minus(c2);
System.out.println("c1 - c2 = " + c5.toString());
Complex c6 = c1.multiply(c2);
System.out.println("c1 * c2 = " + c6.toString());
System.out.println("c1.equals(c2) = " + c1.equals(c2));
System.out.println("c1.equals(new Complex(1.0, 2.0)) = " + c1.equals(new Complex(1.0, 2.0)));
System.out.println("|c1| = " + c1.length());
System.out.println("|c2| = " + c2.length());
System.out.println("|c3| = " + c3.length());
}
}
```
在main方法中,我们按照要求使用了实际数据来测试了成员方法。
相关推荐
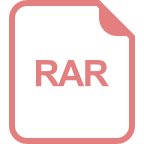
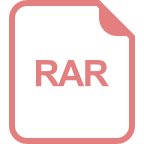
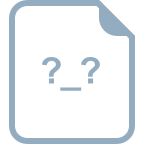














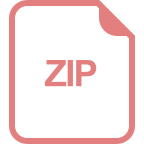