声明复数类,成员变量包括实部和虚部,成员方法包括实现复数加法(实现两个方法, 一个是直接在原来的复数上加;另一个生成新的复数),减法(同加法)、字符串描述(toString 方法)、比较两个复数是否相等等操作。编写 Java 程序创建对象及测试其方法的功能。
时间: 2024-09-28 12:09:37 浏览: 52
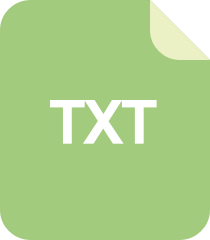
java .定义一个复数类 complex ,它的内部具有两个实例变量:realPart和imagPart,分别代表复数的实部和虚

在Java中,你可以创建一个名为ComplexNumber的类来表示复数,包含成员变量real和imaginary分别代表实部和虚部,并提供相应的操作方法。以下是这个类的示例代码:
```java
public class ComplexNumber {
private double real;
private double imaginary;
// 构造函数
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 成员方法:复数加法(直接修改原对象)
public void add(ComplexNumber other) {
real += other.real;
imaginary += other.imaginary;
}
// 另一种加法方法(返回新复数)
public ComplexNumber addNew(ComplexNumber other) {
return new ComplexNumber(real + other.real, imaginary + other.imaginary);
}
// 减法方法(同加法,可以添加对应版本)
public void subtract(ComplexNumber other) {
real -= other.real;
imaginary -= other.imaginary;
}
public ComplexNumber subtractNew(ComplexNumber other) {
return new ComplexNumber(real - other.real, imaginary - other.imaginary);
}
// 字符串描述方法
@Override
public String toString() {
return "ComplexNumber{" +
"real=" + real +
", imaginary=" + imaginary +
'}';
}
// 比较两个复数是否相等
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
ComplexNumber complexNum = (ComplexNumber) obj;
return Double.compare(real, complexNum.real) == 0 &&
Double.compare(imaginary, complexNum.imaginary) == 0;
}
}
// 测试部分
public class Main {
public static void main(String[] args) {
ComplexNumber c1 = new ComplexNumber(2, 3);
ComplexNumber c2 = new ComplexNumber(4, 5);
System.out.println(c1); // 输出初始的复数
// 直接在c1上加c2
c1.add(c2);
System.out.println(c1); // 打印加后的复数
// 创建一个新的复数并相加
ComplexNumber result = c1.addNew(c2);
System.out.println(result.toString());
// 测试减法
c1.subtract(c2);
System.out.println(c1);
// 使用equals方法比较两个复数是否相等
System.out.println(c1.equals(new ComplexNumber(6, 8)) ? "相等" : "不相等");
}
}
```
在这个程序中,我们创建了一个复数类并实现了基本的操作。在`main`方法中,我们创建了两个复数对象,进行了加法和减法运算,并验证了相等性。
阅读全文
相关推荐
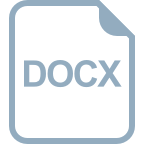
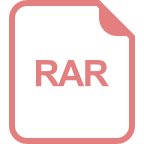














