qt 使用libcurl发送请求,和flask服务器进行通信
时间: 2023-08-21 22:05:47 浏览: 138
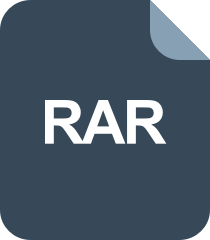
qt下libcurl的封装和使用(包含库封装、库调用、http服务器)
你可以使用libcurl库在Qt中发送HTTP请求,与Flask服务器进行通信。以下是一个简单的示例代码:
```c++
#include <QCoreApplication>
#include <QDebug>
#include <QUrl>
#include <curl/curl.h>
// 回调函数,用于处理curl请求的响应数据
size_t handle_response(char *buffer, size_t size, size_t nmemb, void *userdata)
{
QByteArray *response = static_cast<QByteArray*>(userdata);
response->append(buffer, size * nmemb);
return size * nmemb;
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 初始化curl
curl_global_init(CURL_GLOBAL_ALL);
CURL *curl = curl_easy_init();
// 设置请求的URL
QUrl url("http://localhost:5000/api/test");
// 设置curl选项
curl_easy_setopt(curl, CURLOPT_URL, url.toString().toStdString().c_str());
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L);
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, handle_response);
// 发送请求
QByteArray response;
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &response);
CURLcode result = curl_easy_perform(curl);
if (result != CURLE_OK) {
qDebug() << "Error: " << curl_easy_strerror(result);
}
else {
qDebug() << "Response: " << response;
}
// 清理curl
curl_easy_cleanup(curl);
curl_global_cleanup();
return a.exec();
}
```
在上面的示例中,我们使用了`curl_easy_setopt`函数设置了curl选项,包括请求的URL、是否跟随重定向、响应数据的处理函数等。我们使用了`curl_easy_perform`函数发送请求并获取响应数据,最后使用`curl_easy_cleanup`函数清理curl。
阅读全文
相关推荐
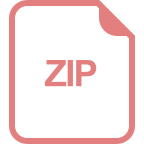
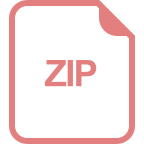
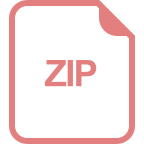
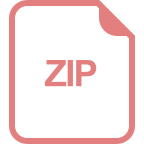
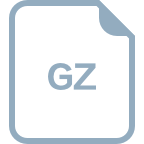
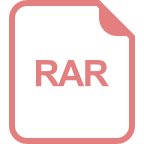
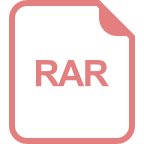
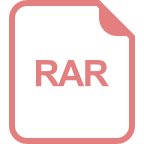
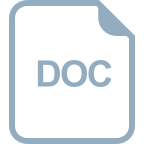
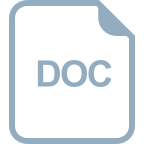
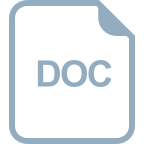






