qt libcurl发送put
时间: 2023-09-04 13:17:20 浏览: 98
以下是使用Qt和libcurl发送PUT请求的示例代码:
```
#include <QtCore/QCoreApplication>
#include <curl/curl.h>
// This function will be called by libcurl when it has data to send
static size_t write_callback(void *ptr, size_t size, size_t nmemb, void *userdata)
{
// Here you can handle the response from the server
return size * nmemb;
}
int main(int argc, char *argv[])
{
QCoreApplication app(argc, argv);
// Initialize libcurl
curl_global_init(CURL_GLOBAL_ALL);
// Create a new curl handle
CURL *curl = curl_easy_init();
if (!curl) {
// Handle error
return -1;
}
// Set the URL for the request
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com/your-resource");
// Set the HTTP method to PUT
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, "PUT");
// Set the data to send in the request body
const char *data = "Hello, world!";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, data);
// Set the size of the data to send
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, strlen(data));
// Set the callback function to handle the response from the server
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_callback);
// Perform the request
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
// Handle error
}
// Clean up
curl_easy_cleanup(curl);
curl_global_cleanup();
return app.exec();
}
```
相关推荐
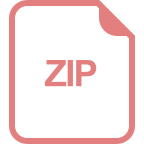
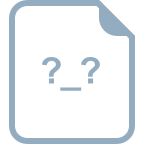
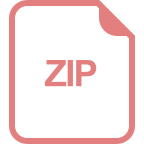














