qt libcurl发送post表单图片
时间: 2023-12-23 12:04:17 浏览: 107
以下是使用Qt和libcurl发送POST表单图片的示例代码:
```c++
#include <QtNetwork>
#include <curl/curl.h>
void sendPostFormImage() {
// 1. 读取图片数据
QFile file("image.jpg");
if (!file.open(QIODevice::ReadOnly)) {
qDebug() << "Failed to open file";
return;
}
QByteArray imageData = file.readAll();
file.close();
// 2. 设置POST表单数据
QString boundary = "---------------------------" + QString::number(QDateTime::currentMSecsSinceEpoch());
QByteArray postData;
postData.append("--" + boundary + "\r\n");
postData.append("Content-Disposition: form-data; name=\"image\"; filename=\"image.jpg\"\r\n");
postData.append("Content-Type: image/jpeg\r\n\r\n");
postData.append(imageData + "\r\n");
postData.append("--" + boundary + "--\r\n");
// 3. 设置libcurl参数
CURL* curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com/upload");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postData.data());
curl_easy_setopt(curl, CURLOPT_POSTFIELDSIZE, postData.size());
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, QStringList() << "Content-Type: multipart/form-data; boundary=" + boundary.toLatin1().data());
curl_easy_setopt(curl, CURLOPT_USERAGENT, "libcurl-agent/1.0");
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1L);
// 4. 执行请求
CURLcode res = curl_easy_perform(curl);
if (res != CURLE_OK) {
qDebug() << "Failed to send POST form: " << curl_easy_strerror(res);
}
curl_easy_cleanup(curl);
}
}
```
该示例使用Qt的QFile类读取图片数据,然后使用libcurl发送POST表单数据,其中包括一个包含图片数据的文件字段。boundary变量用于分隔不同的表单字段。最后,将Content-Type标头设置为"multipart/form-data"和boundary值,以便服务器正确解析数据。
阅读全文
相关推荐
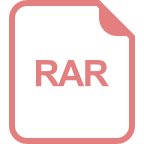








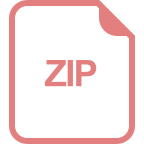
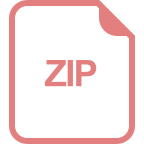
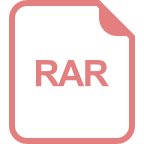





