编写卷积神经网络人脸识别代码
时间: 2023-07-20 12:29:02 浏览: 97
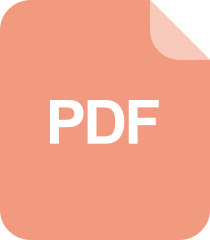
使用卷积神经网络(CNN)做人脸识别的示例代码
好的,下面是一个简单的卷积神经网络人脸识别的代码,使用的是Python和Keras框架。
首先,需要导入相关的库和模块:
```python
import numpy as np
import keras
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten
from keras.layers import Conv2D, MaxPooling2D
from keras.optimizers import Adam
from keras.preprocessing.image import ImageDataGenerator
```
接着,需要设置一些超参数,如图像的尺寸、batch size、epoch等:
```python
img_width, img_height = 48, 48
batch_size = 32
epochs = 50
```
然后,可以定义一个卷积神经网络模型,包括卷积层、池化层和全连接层等:
```python
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(img_width, img_height, 1)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(128, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(64, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(7, activation='softmax'))
```
在定义模型后,需要编译模型,并设置一些参数:
```python
model.compile(loss='categorical_crossentropy',
optimizer=Adam(lr=0.0001, decay=1e-6),
metrics=['accuracy'])
```
接着,需要对数据进行预处理和增强,可以使用Keras提供的ImageDataGenerator:
```python
train_datagen = ImageDataGenerator(
rescale=1./255,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True)
test_datagen = ImageDataGenerator(rescale=1./255)
train_generator = train_datagen.flow_from_directory(
'train',
color_mode='grayscale',
target_size=(img_width, img_height),
batch_size=batch_size,
class_mode='categorical')
validation_generator = test_datagen.flow_from_directory(
'test',
color_mode='grayscale',
target_size=(img_width, img_height),
batch_size=batch_size,
class_mode='categorical')
```
最后,可以使用fit_generator对模型进行训练:
```python
model.fit_generator(
train_generator,
steps_per_epoch=train_generator.n // batch_size,
epochs=epochs,
validation_data=validation_generator,
validation_steps=validation_generator.n // batch_size)
```
以上就是一个简单的卷积神经网络人脸识别的代码,当然还可以根据具体的需求进行一些修改和优化。
阅读全文
相关推荐
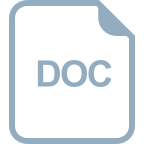
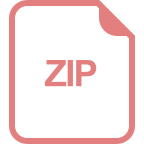
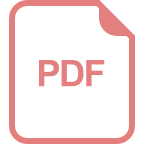
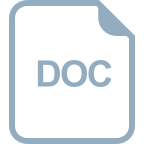


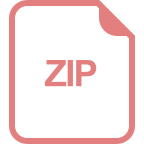
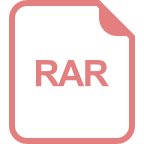
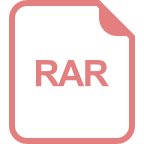
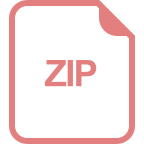
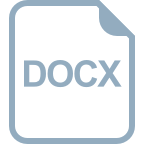
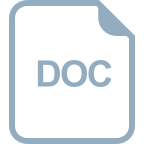
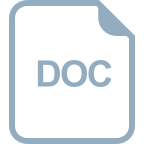
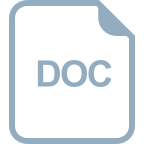
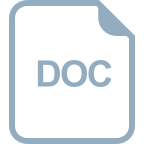
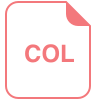