uniapp实现白色背景,内部圆环进度条,进度有渐进动画,百分比由参数控制的vue组件
时间: 2024-03-12 19:44:58 浏览: 30
可以通过自定义组件的方式,在 UniApp 中实现白色背景、内部圆环进度条、进度有渐进动画、百分比由参数控制的效果。
以下是一个简单的实现示例:
1. 在 components 目录下创建一个名为 "circle-progress" 的文件夹,并在该文件夹下创建一个 "circle-progress.vue" 的组件文件。
2. 在 "circle-progress.vue" 中定义组件的模板和样式,如下所示:
```html
<template>
<view class="circle-progress">
<view class="circle-progress-bg"></view>
<view class="circle-progress-fore" :style="progressStyle"></view>
<view class="circle-progress-text">{{ percentage }}%</view>
</view>
</template>
<style scoped>
.circle-progress {
position: relative;
width: 100px;
height: 100px;
border-radius: 50%;
background-color: #fff;
overflow: hidden;
}
.circle-progress-bg {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
border-radius: 50%;
border: 1px solid #ccc;
}
.circle-progress-fore {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
border-radius: 50%;
border: 1px solid #fff;
clip: rect(0, 50px, 100px, 0);
transform-origin: center center;
animation: progress 1s ease forwards;
}
.circle-progress-text {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
font-size: 16px;
font-weight: bold;
color: #333;
}
</style>
```
3. 在组件的 script 标签中定义组件的 props 和 computed 属性,以及计算进度条样式的方法,如下所示:
```html
<script>
export default {
props: {
percentage: {
type: Number,
required: true,
validator: (val) => val >= 0 && val <= 100,
},
},
computed: {
progressStyle() {
return {
transform: `rotate(${(this.percentage / 100) * 360}deg)`,
};
},
},
};
</script>
```
4. 在父组件中引用和使用自定义的 "circle-progress" 组件,并传递需要展示的百分比值,如下所示:
```html
<template>
<view class="container">
<circle-progress :percentage="progress"></circle-progress>
</view>
</template>
<script>
import CircleProgress from "@/components/circle-progress/circle-progress.vue";
export default {
components: {
CircleProgress,
},
data() {
return {
progress: 0,
};
},
mounted() {
this.animateProgress();
},
methods: {
animateProgress() {
let current = 0;
const end = 80;
const step = 1;
const interval = setInterval(() => {
current += step;
this.progress = current;
if (current >= end) {
clearInterval(interval);
}
}, 10);
},
},
};
</script>
```
在父组件中通过 animateProgress 方法模拟进度条的渐进动画,当进度条达到指定的值时,清除定时器并停止动画。
以上示例仅供参考,具体实现方式和样式可以根据需要进行调整。
相关推荐
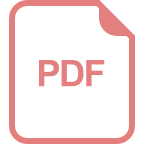
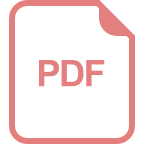
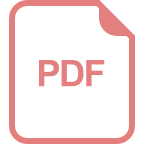
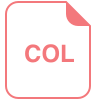
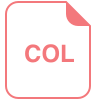
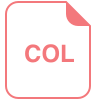











