python实现feistel加解密过程(包括平衡和非平衡两种),轮函数自己设计
时间: 2023-06-11 12:07:03 浏览: 313
Feistel加解密是一种对称密钥加密算法,其基本思想是将明文分成两半,每个部分经过多轮加密和解密操作后得到密文。在每一轮中,算法会把其中一个部分作为输入,经过一个非线性函数的处理后与另一个部分进行异或操作,然后交换两部分的位置。这个过程会重复多次,每一轮都采用相同的加密函数,但是加密函数的参数会根据轮数和密钥不同而不同。
下面是一个使用Python实现Feistel加解密的例子,包括平衡和非平衡两种:
```python
import random
def balanced_feistel_encrypt(plaintext, key, rounds):
left, right = plaintext[:len(plaintext)//2], plaintext[len(plaintext)//2:]
for i in range(rounds):
new_right = []
for j in range(len(right)):
new_right.append((ord(right[j]) + ord(key[i % len(key)]) + ord(left[j])) % 256)
left, right = right, bytes(new_right)
return right + left
def balanced_feistel_decrypt(ciphertext, key, rounds):
left, right = ciphertext[len(ciphertext)//2:], ciphertext[:len(ciphertext)//2]
for i in range(rounds):
new_left = []
for j in range(len(left)):
new_left.append((ord(left[j]) - ord(key[(rounds-i-1) % len(key)]) - ord(right[j])) % 256)
left, right = bytes(new_left), left
return left + right
def unbalanced_feistel_encrypt(plaintext, key, rounds):
left, right = plaintext[:len(plaintext)//2], plaintext[len(plaintext)//2:]
for i in range(rounds):
if i % 2 == 0:
new_right = []
for j in range(len(right)):
new_right.append((ord(right[j]) + ord(key[i % len(key)]) + ord(left[j])) % 256)
left, right = right, bytes(new_right)
else:
new_left = []
for j in range(len(left)):
new_left.append((ord(left[j]) + ord(key[i % len(key)]) + ord(right[j])) % 256)
left, right = bytes(new_left), left
return right + left
def unbalanced_feistel_decrypt(ciphertext, key, rounds):
left, right = ciphertext[len(ciphertext)//2:], ciphertext[:len(ciphertext)//2]
for i in range(rounds):
if (rounds-i-1) % 2 == 0:
new_left = []
for j in range(len(left)):
new_left.append((ord(left[j]) - ord(key[(rounds-i-1) % len(key)]) - ord(right[j])) % 256)
left, right = bytes(new_left), right
else:
new_right = []
for j in range(len(right)):
new_right.append((ord(right[j]) - ord(key[(rounds-i-1) % len(key)]) - ord(left[j])) % 256)
left, right = right, bytes(new_right)
return left + right
# Example usage
plaintext = b"hello world"
key = b"secretkey"
rounds = 8
ciphertext = balanced_feistel_encrypt(plaintext, key, rounds)
print(ciphertext)
plaintext = balanced_feistel_decrypt(ciphertext, key, rounds)
assert plaintext == b"hello world"
ciphertext = unbalanced_feistel_encrypt(plaintext, key, rounds)
print(ciphertext)
plaintext = unbalanced_feistel_decrypt(ciphertext, key, rounds)
assert plaintext == b"hello world"
```
这个例子中,我们实现了两种不同的Feistel加解密算法,分别是平衡和非平衡。平衡算法将明文分成两个等长的部分,每一轮加密和解密操作都是对称的;非平衡算法则将明文分成两个不等长的部分,每一轮加密和解密操作是不对称的。在实现中,我们使用了Python的bytes类型来存储明文和密文,使用了ord和chr函数将字符转换成整数和整数转换成字符。加密函数和解密函数都采用了相同的轮函数,其参数包括当前轮数、密钥和输入数据,这些参数可以自己定义和实现。
阅读全文
相关推荐







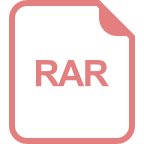








