Python实现Feistel加解密过程
时间: 2023-11-05 20:06:44 浏览: 101
Feistel加解密是一种对称加密算法,它通过将明文分成两部分,交替进行加密和解密的过程,最终得到密文。下面是一个使用Python实现Feistel加解密的简单例子。
```python
import binascii
# Feistel加密函数
def feistel_encrypt(text, key, rounds):
# 将明文转换为二进制
text = bin(int(binascii.hexlify(text.encode()), 16))[2:]
# 将密钥转换为二进制
key = bin(int(binascii.hexlify(key.encode()), 16))[2:]
# 将明文填充到偶数位
if len(text) % 2 != 0:
text = text + '0'
# 将密钥填充到偶数位
if len(key) % 2 != 0:
key = key + '0'
# 将明文分成左右两部分
left = text[:len(text) // 2]
right = text[len(text) // 2:]
# 进行指定轮数的加密
for i in range(rounds):
new_right = int(left, 2) ^ int(key, 2)
new_right = '{0:b}'.format(new_right).zfill(len(right))
new_right = int(new_right, 2) ^ int(right, 2)
left, right = right, new_right
# 将加密后的左右两部分合并为密文
result = left + right
# 将密文转换为ASCII字符
result = binascii.unhexlify('%x' % int(result, 2)).decode('utf-8')
return result
# Feistel解密函数
def feistel_decrypt(cipher, key, rounds):
# 将密文转换为二进制
cipher = bin(int(binascii.hexlify(cipher.encode()), 16))[2:]
# 将密钥转换为二进制
key = bin(int(binascii.hexlify(key.encode()), 16))[2:]
# 将密文分成左右两部分
left = cipher[:len(cipher) // 2]
right = cipher[len(cipher) // 2:]
# 进行指定轮数的解密
for i in range(rounds):
new_left = int(right, 2) ^ int(key, 2)
new_left = '{0:b}'.format(new_left).zfill(len(left))
new_left = int(new_left, 2) ^ int(left, 2)
left, right = new_left, left
# 将解密后的左右两部分合并为明文
result = left + right
# 将明文转换为ASCII字符
result = binascii.unhexlify('%x' % int(result, 2)).decode('utf-8')
return result
# 测试加解密过程
text = 'Hello, world!'
key = '123456'
rounds = 16
cipher = feistel_encrypt(text, key, rounds)
print('加密后的密文:', cipher)
result = feistel_decrypt(cipher, key, rounds)
print('解密后的明文:', result)
```
在上面的代码中,我们使用了Python的binascii库将字符串转换成二进制,然后根据Feistel加密的过程,分别实现了加密和解密的函数。最后,我们测试了加解密过程,可以看到,经过加密和解密之后,明文和密文是一致的。
相关推荐
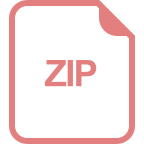
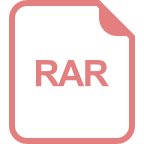















