根据以上要求帮我写一段完整的代码
时间: 2024-04-08 14:29:17 浏览: 26
以下是一个简单的面向过程的C++图书馆管理系统的代码示例:
```cpp
#include <iostream>
#include <vector>
#include <string>
struct Book {
std::string title;
std::string author;
std::string publicationDate;
bool isAvailable;
};
std::vector<Book> library;
void addBook() {
Book book;
std::cout << "Enter book title: ";
std::cin >> book.title;
std::cout << "Enter book author: ";
std::cin >> book.author;
std::cout << "Enter publication date: ";
std::cin >> book.publicationDate;
book.isAvailable = true;
library.push_back(book);
std::cout << "Book added successfully!" << std::endl;
}
void deleteBook() {
std::string title;
std::cout << "Enter book title to delete: ";
std::cin >> title;
for (auto it = library.begin(); it != library.end(); ++it) {
if ((*it).title == title) {
library.erase(it);
std::cout << "Book deleted successfully!" << std::endl;
return;
}
}
std::cout << "Book not found!" << std::endl;
}
void displayBooks() {
if (library.empty()) {
std::cout << "No books in the library." << std::endl;
return;
}
for (const auto& book : library) {
std::cout << "Title: " << book.title << std::endl;
std::cout << "Author: " << book.author << std::endl;
std::cout << "Publication Date: " << book.publicationDate << std::endl;
std::cout << "Availability: " << (book.isAvailable ? "Available" : "Not Available") << std::endl;
std::cout << "----------------------" << std::endl;
}
}
void menu() {
std::cout << "Library Management System" << std::endl;
std::cout << "1. Add Book" << std::endl;
std::cout << "2. Delete Book" << std::endl;
std::cout << "3. Display Books" << std::endl;
std::cout << "4. Quit" << std::endl;
std::cout << "Enter your choice: ";
}
int main() {
int choice;
while (true) {
menu();
std::cin >> choice;
switch (choice) {
case 1:
addBook();
break;
case 2:
deleteBook();
break;
case 3:
displayBooks();
break;
case 4:
std::cout << "Quitting the program..." << std::endl;
return 0;
default:
std::cout << "Invalid choice. Please try again." << std::endl;
break;
}
std::cout << std::endl;
}
return 0;
}
```
这段代码实现了添加图书、删除图书、显示图书等基本功能。你可以根据需要进行修改和扩展,例如增加借阅和归还图书的功能。请确保在运行代码之前已经创建好了C++项目,并将以上代码复制到项目文件中进行编译和运行。
相关推荐
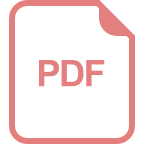














