type 'class array' argument given to 'delete', expected pointer
时间: 2024-01-03 16:03:46 浏览: 322
This error occurs when you try to delete an array using the `delete` operator with the argument of `class array`, instead of a pointer to the array. The `delete` operator is used to deallocate memory that was previously allocated using the `new` operator.
To fix this error, make sure you pass a pointer to the array when using the `delete` operator. For example:
```
int* myArray = new int[10]; // allocate memory for an array of 10 integers
delete[] myArray; // deallocate memory using the delete[] operator with a pointer to the array
```
Note the use of `delete[]` instead of `delete` when deallocating an array, as it ensures that all elements in the array are properly deallocated.
相关问题
回答代码报错main.c:12:19: error: type 'class Abc' argument given to 'delete', expected pointer delete [] a[2]; ^
抱歉,我之前的回答有误。对于你所描述的情况,`a`是一个指向`Abc`类对象数组的指针,因此需要使用`delete[]`来释放整个数组。而不能像释放单个对象那样使用`delete`。
下面是正确的代码示例:
```cpp
delete [] a;
```
这将释放整个`a`数组,包括所有的`Abc`对象。请注意,这样做会删除整个数组,而不仅仅是第三个元素。如果你只想删除第三个元素,那么需要将其他元素移动到合适的位置。
/home/cps/CPS_Project/CPS/src/app/ConfigurationManager/DBConfig_manager.cpp:137: error: type ‘class QProcess’ argument given to ‘delete’, expected pointer delete process; ^
这个错误通常是因为你试图删除一个非指针类型的对象。`delete` 关键字只能用于指针类型的对象,而 `QProcess` 是一个类类型,不是一个指针类型。要解决这个错误,你需要修改代码,确保你只删除指针类型的对象。你可以尝试使用 `QScopedPointer` 或 `std::unique_ptr` 等智能指针来管理对象的生命周期,以避免手动释放内存和删除对象的问题。例如:
```
QScopedPointer<QProcess> process(new QProcess);
// do something with process
process->terminate();
process->waitForFinished();
// process will be deleted automatically when it goes out of scope
```
或者:
```
std::unique_ptr<QProcess> process(new QProcess);
// do something with process
process->terminate();
process->waitForFinished();
// process will be deleted automatically when it goes out of scope
```
阅读全文
相关推荐




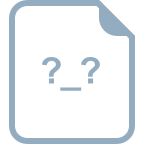



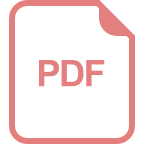






