用verilog代码写一个VGA输出模块
时间: 2023-10-25 14:09:49 浏览: 92
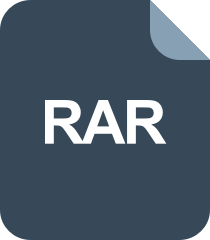
基于verilog的VGA程序

以下是一个简单的Verilog代码实现:
```
module vga_output(
input clk,
input [9:0] h_count,
input [8:0] v_count,
output reg [3:0] red,
output reg [3:0] green,
output reg [3:0] blue,
output reg h_sync,
output reg v_sync
);
// VGA timing parameters
parameter H_FRONT_PORCH = 16;
parameter H_SYNC_WIDTH = 96;
parameter H_BACK_PORCH = 48;
parameter H_DISPLAY = 640;
parameter V_FRONT_PORCH = 10;
parameter V_SYNC_WIDTH = 2;
parameter V_BACK_PORCH = 33;
parameter V_DISPLAY = 480;
// Horizontal counters
reg [3:0] h_sync_count = 0;
reg [3:0] h_back_porch_count = 0;
reg [3:0] h_display_count = 0;
reg [3:0] h_front_porch_count = 0;
// Vertical counters
reg [3:0] v_sync_count = 0;
reg [3:0] v_back_porch_count = 0;
reg [3:0] v_display_count = 0;
reg [3:0] v_front_porch_count = 0;
// Sync signals
reg h_sync = 1;
reg v_sync = 1;
// Color signals
reg [3:0] red = 0;
reg [3:0] green = 0;
reg [3:0] blue = 0;
always @(posedge clk) begin
// Horizontal counters
if (h_sync_count < H_SYNC_WIDTH) begin
h_sync_count <= h_sync_count + 1;
h_sync <= 0;
end else if (h_back_porch_count < H_BACK_PORCH) begin
h_back_porch_count <= h_back_porch_count + 1;
h_sync <= 0;
end else if (h_display_count < H_DISPLAY) begin
h_display_count <= h_display_count + 1;
red <= // your red color value here
green <= // your green color value here
blue <= // your blue color value here
h_sync <= 1;
end else if (h_front_porch_count < H_FRONT_PORCH) begin
h_front_porch_count <= h_front_porch_count + 1;
h_sync <= 0;
end else begin
h_sync_count <= 0;
h_back_porch_count <= 0;
h_display_count <= 0;
h_front_porch_count <= 0;
end
// Vertical counters
if (v_sync_count < V_SYNC_WIDTH) begin
v_sync_count <= v_sync_count + 1;
v_sync <= 0;
end else if (v_back_porch_count < V_BACK_PORCH) begin
v_back_porch_count <= v_back_porch_count + 1;
v_sync <= 0;
end else if (v_display_count < V_DISPLAY) begin
v_display_count <= v_display_count + 1;
v_sync <= 1;
end else if (v_front_porch_count < V_FRONT_PORCH) begin
v_front_porch_count <= v_front_porch_count + 1;
v_sync <= 0;
end else begin
v_sync_count <= 0;
v_back_porch_count <= 0;
v_display_count <= 0;
v_front_porch_count <= 0;
end
end
endmodule
```
该代码实现了一个名为`vga_output`的模块,该模块使用VGA标准将颜色输出到显示器。该模块包含时钟输入`clk`,水平和垂直计数器输入`h_count`和`v_count`,以及红色、绿色和蓝色输出端口`red`、`green`和`blue`,以及水平和垂直同步输出端口`h_sync`和`v_sync`。
在始终块中,我们使用计数器来跟踪当前扫描线和帧位置,并根据VGA时序生成水平和垂直同步信号。我们还使用计数器和颜色值生成三个颜色信号。请注意,此代码中使用的VGA时序参数是示例值,您可以根据需要自行调整这些值。同时,您需要将`red`、`green`和`blue`的赋值语句替换为您的颜色值计算代码。
阅读全文
相关推荐
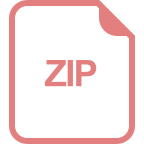
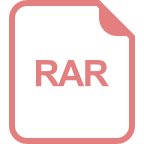






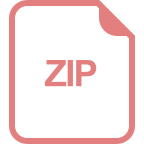
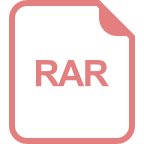
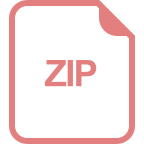
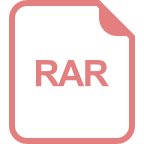
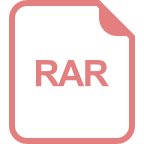
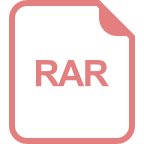
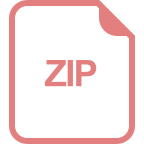
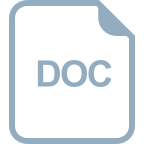