cin >> list->alumni[list->Stu_length].ID;这段代码显示[Error] no match for 'operator>>' (operand types are 'std::istream {aka std::basic_istream<char>}' and 'int [20]') 和[Error] candidates are: 这两个错误,请改正
时间: 2024-02-13 07:04:56 浏览: 131
这段代码的问题在于,你正在尝试将输入流中的数据读取到一个整型数组中,但输入运算符(>>)不支持将数据读取到数组中。正确的做法是将输入流中的数据读取到单个整数中,然后将该整数存储到数组的一个位置中。修改代码如下:
```cpp
cin >> list->alumni[list->Stu_length].ID[0]; // 读取一个整数到数组的第一个位置中
```
这样可以解决上述错误。当然,如果你需要读取多个整数到数组中,可以使用循环来逐个读取。
相关问题
strcpy(cf_dept.name, "护理学院"); cf_dept.alumni_list = (struct alumni*)malloc(sizeof(struct alumni) * 60); cf_dept.alumni_count = 0; cf_dept.sub_departments = (struct department*)malloc(sizeof(struct department) * 60); cf_dept.sub_dept_count = 0;这段代码显示[Error] 'cf_dept' was not declared in this scope 的错误,请改正
这段代码中使用了一个名为 "cf_dept" 的变量,但是没有先进行声明。为了解决这个问题,我们需要在代码的前面先声明 "cf_dept" 变量的类型和名称,例如:
```c++
struct department cf_dept;
```
这样就可以在后续的代码中使用 "cf_dept" 变量了。完整的代码如下所示:
```c++
#include <stdlib.h>
#include <string.h>
struct alumni {
char name[20];
int age;
};
struct department {
char name[20];
struct alumni* alumni_list;
int alumni_count;
struct department* sub_departments;
int sub_dept_count;
};
int main() {
struct department cf_dept;
strcpy(cf_dept.name, "护理学院");
cf_dept.alumni_list = (struct alumni*)malloc(sizeof(struct alumni) * 60);
cf_dept.alumni_count = 0;
cf_dept.sub_departments = (struct department*)malloc(sizeof(struct department) * 60);
cf_dept.sub_dept_count = 0;
return 0;
}
```
// 创建一个新的校友信息节点 Alumni *create_alumni(char *name, int grad_year, char *phone) { Alumni *alumni = (Alumni *)malloc(sizeof(Alumni)); strcpy(alumni->name, name); alumni->grad_year = grad_year; strcpy(alumni->phone, phone); alumni->dept = NULL; return alumni; } // 创建一个新的院系节点 Department *create_department(char *name) { Department *dept = (Department *)malloc(sizeof(Department)); strcpy(dept->name, name); dept->alumni_list = NULL; dept->left = NULL; dept->right = NULL; return dept; }这段代码显示initializing argument 1 of 'Alumni* create_alumni(char*, int, char*)'和initializing argument 1 of 'Department* create_department(char*)'这两个错误,请改正
错误提示表明函数调用时传入的第一个参数类型与函数定义时的类型不匹配。具体来说,函数声明中第一个参数需要的是指向字符数组的指针,而调用时传入的是字符数组本身。
为了解决这个问题,我们需要修改函数调用时的参数。将需要传入的字符数组改为指向字符数组的指针即可。修改后的代码如下:
```
// 创建一个新的校友信息节点
Alumni *create_alumni(char *name, int grad_year, char *phone) {
Alumni *alumni = (Alumni *)malloc(sizeof(Alumni));
strcpy(alumni->name, name);
alumni->grad_year = grad_year;
strcpy(alumni->phone, phone);
alumni->dept = NULL;
return alumni;
}
// 创建一个新的院系节点
Department *create_department(char *name) {
Department *dept = (Department *)malloc(sizeof(Department));
strcpy(dept->name, name);
dept->alumni_list = NULL;
dept->left = NULL;
dept->right = NULL;
return dept;
}
int main() {
// 修改调用参数为指向字符数组的指针
Alumni *alumni = create_alumni("张三", 2021, "1234567890");
Department *dept = create_department("计算机科学与技术");
return 0;
}
```
阅读全文
相关推荐
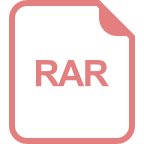
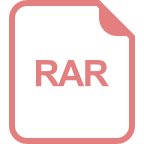
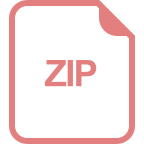
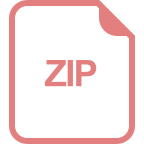
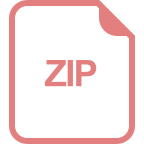
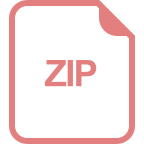
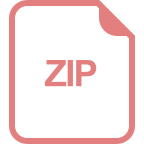
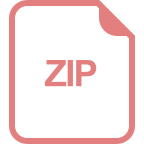
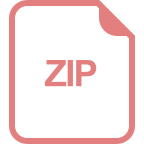
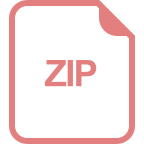
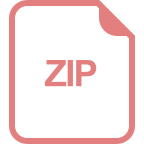
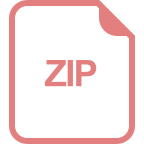
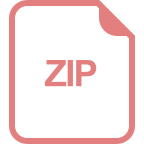
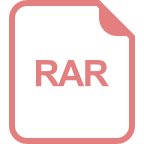
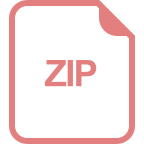