std::next_permutation
时间: 2024-04-30 20:16:09 浏览: 21
std::next_permutation是一个STL算法,它可以将容器中的元素重新排列为下一个字典序排列。如果容器中的元素已经是字典序最大的排列,则next_permutation返回false,否则它将重新排列容器中的元素,并返回true。具体实现可以参考下面的代码:
```
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
do {
for (auto elem : vec) {
std::cout << elem << " ";
}
std::cout << std::endl;
} while (std::next_permutation(vec.begin(), vec.end()));
return 0;
}
```
上述代码中的do-while循环会输出{1,2,3}的所有字典序排列,即:
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
相关问题
"next_permutation": 不是 "std" 的成员
"next_permutation" 是一个算法函数,用于生成给定序列的下一个排列。它不是 "std" 的成员函数,而是属于 C++ 标准库的<algorithm>头文件中的函数。
该函数接受两个迭代器作为参数,表示要生成排列的范围。它会将范围内的元素重新排列为下一个字典序更大的排列,并返回 true。如果已经是最后一个排列,则会将范围内的元素重新排列为第一个排列,并返回 false。
下面是 "next_permutation" 函数的使用示例:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
do {
for (int num : nums) {
std::cout << num << " ";
}
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end()));
return 0;
}
```
输出结果为:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
next_permutation
next_permutation is a function in the C++ STL (Standard Template Library) that generates the next lexicographically greater permutation of a sequence. It modifies the sequence in place, and if there is no greater permutation, it returns false.
Here is an example usage of next_permutation:
```
#include <algorithm>
#include <iostream>
#include <vector>
int main() {
std::vector<int> v = {1, 2, 3};
do {
for (int i : v) {
std::cout << i << ' ';
}
std::cout << '\n';
} while (std::next_permutation(v.begin(), v.end()));
return 0;
}
```
This program generates all permutations of the sequence {1, 2, 3}:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
The function std::next_permutation is called in the while loop condition to generate the next permutation. The loop continues until there is no greater permutation left.
相关推荐
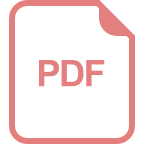
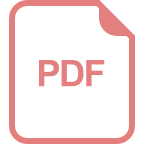
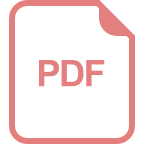












