c++next_permutation用法
时间: 2023-04-24 12:05:56 浏览: 108
c++中的next_permutation函数用于将一个序列变为下一个排列。具体来说,它会将当前序列重新排列为下一个字典序更大的序列,如果已经是最大的排列,则返回false。
使用方法:
1. 首先需要包含头文件<algorithm>
2. next_permutation函数的参数是一个迭代器,表示要排列的序列的起始位置和结束位置。
3. 调用next_permutation函数后,会将当前序列重新排列为下一个字典序更大的序列,如果已经是最大的排列,则返回false。
示例代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
int arr[] = {1, 2, 3};
do {
for (int i = 0; i < 3; i++) {
cout << arr[i] << " ";
}
cout << endl;
} while (next_permutation(arr, arr + 3));
return 0;
}
```
输出结果:
```
1 2 3
1 3 2
2 1 3
2 3 1
3 1 2
3 2 1
```
以上代码将数组{1, 2, 3}的所有排列输出了出来。
相关问题
c++中next_permutation用法
`std::next_permutation` 是 C++ 标准库 `<algorithm>` 中的一个函数,它用于生成序列的下一个排列。这个函数通常用在需要对容器中的元素进行排序并寻找所有可能排列的场景,比如回溯算法或者一些游戏中涉及随机排列的情况。
该函数接受两个迭代器,分别指向待操作的范围的第一个和最后一个元素。它会将当前范围内的元素按递增顺序排列,然后找到第一个比它后面的元素小的元素,并将其与其右侧的最大元素交换,使得整个序列变成一个新的递增排列。如果序列已经是最大的,则表示没有更多的排列,此时函数返回 `false`;否则,返回 `true`,并且更新后的序列已经变为下一个排列。
下面是一个简单的示例:
```cpp
#include <iostream>
#include <algorithm>
void print_permutation(std::vector<int>& nums) {
std::sort(nums.begin(), nums.end()); // 先排序
do {
for (const auto& num : nums)
std::cout << num << ' ';
std::cout << '\n';
} while (std::next_permutation(nums.begin(), nums.end()));
}
int main() {
std::vector<int> nums = {1, 2, 3};
print_permutation(nums);
return 0;
}
```
在这个例子中,`print_permutation` 函数会打印出给定整数向量的所有排列。
c++中next_permutation函数用法
next_permutation函数是C++ STL中的一个函数,用于生成下一个排列。它接受两个迭代器作为参数,表示排列的起始和结束位置。如果存在下一个排列,则函数返回true,并将排列修改为下一个排列;否则,函数返回false,并将排列修改为第一个排列。
阅读全文
相关推荐
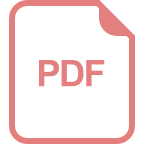
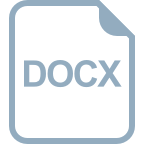













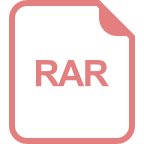
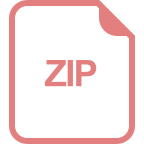