python提取文件名多个关键字查找文件夹内是否存在并打印
时间: 2024-12-19 22:22:21 浏览: 10
在Python中,你可以使用`os`和`fnmatch`模块来遍历文件夹并查找包含特定关键字的文件名。以下是一个简单的示例,展示如何提取文件名中包含多个关键字的文件:
```python
import os
import fnmatch
def search_files(directory, keywords):
# 指定要搜索的目录
for root, dirs, files in os.walk(directory):
# 遍历每个文件
for file in files:
# 使用fnmatch检查文件名是否匹配所有关键字
if all(fnmatch.fnmatch(file, f'*{keyword}*') for keyword in keywords):
print(f"找到文件:{os.path.join(root, file)}")
# 定义关键词列表
keywords = ['关键字1', '关键字2']
# 搜索当前工作目录下符合条件的文件
search_files('.', keywords)
```
这个脚本会递归地搜索指定的目录`directory`,如果文件名包含了所有的关键字,就会打印出该文件的完整路径。
相关问题
python提取文件名多个关键字查找文件夹内是否存在并打印不存在的
在Python中,你可以使用`os`和`fnmatch`模块来遍历文件夹,搜索包含特定关键字的文件名。首先,安装`fnmatch`库如果尚未安装(通过`pip install fnmatch`),然后可以编写如下的脚本:
```python
import os
import fnmatch
def find_files(directory, keywords):
# 检查目录是否存在
if not os.path.exists(directory):
print(f"Directory {directory} does not exist.")
return
for root, dirs, files in os.walk(directory): # 遍历目录及其所有子目录
for file in files:
# 使用fnmatch匹配文件名中的关键字
for keyword in keywords:
if any(keyword in fnmatch.fnmatch(file, pattern) for pattern in ['*%s*' % keyword, '*%s*' % keyword.lower()]):
print(os.path.join(root, file)) # 打印找到的文件路径
break # 找到第一个匹配就退出循环
# 示例,检查'example_folder'下是否存在关键词 'keyword1', 'keyword2'
keywords = ['keyword1', 'keyword2']
find_files('example_folder', keywords)
# 如果你想只打印不存在的文件,可以添加一个集合来跟踪已找到的文件,并在最后判断
found_files = set()
if not find_files('example_folder', keywords, found_files):
print("The following files were not found:")
for file in found_files:
print(file)
```
在这个脚本中,`fnmatch.fnmatch()`用于模糊匹配文件名,`'*%s*'`将关键字插入通配符模式中。`os.path.join(root, file)`组合了根目录和文件名。
阅读全文
相关推荐
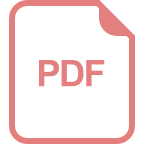
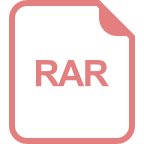
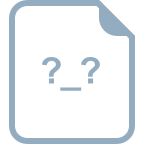
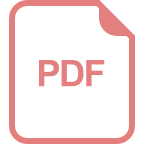
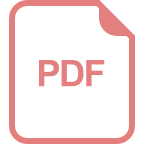
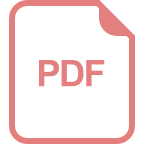
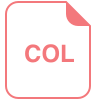
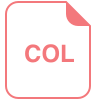
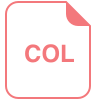
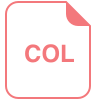
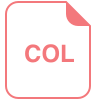
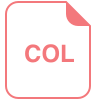
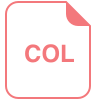
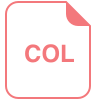
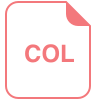
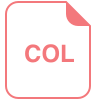