cv2图像锐化算法代码python
时间: 2023-11-10 20:06:12 浏览: 50
根据提供的引用内容,没有直接提到cv2图像锐化算法的代码。但是可以通过引用中提到的四个算法之一——高斯滤波来实现图像的锐化。具体实现代码如下:
```
import cv2
import numpy as np
def sharpen(image):
# 高斯滤波
blurred = cv2.GaussianBlur(image, (0, 0), 3)
# 锐化
sharpened = cv2.addWeighted(image, 1.5, blurred, -0.5, 0)
return sharpened
# 调用函数
img = cv2.imread("image.jpg")
sharpened_img = sharpen(img)
cv2.imshow("Sharpened Image", sharpened_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.GaussianBlur()`函数实现高斯滤波,`cv2.addWeighted()`函数实现图像的加权叠加,从而实现图像的锐化。
相关问题
图像增强算法代码python
以下是几个常用的图像增强算法的Python代码示例:
1. 色彩调整(亮度、对比度、饱和度):
```python
import cv2
def adjust_brightness(image, value):
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
h, s, v = cv2.split(hsv)
v = cv2.add(v, value)
v = np.clip(v, 0, 255)
hsv = cv2.merge((h, s, v))
adjusted = cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)
return adjusted
def adjust_contrast(image, value):
alpha = float(131 + value) / 131
adjusted = cv2.addWeighted(image, alpha, image, 0, 0)
return adjusted
def adjust_saturation(image, value):
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
h, s, v = cv2.split(hsv)
s = cv2.add(s, value)
s = np.clip(s, 0, 255)
hsv = cv2.merge((h, s, v))
adjusted = cv2.cvtColor(hsv, cv2.COLOR_HSV2BGR)
return adjusted
```
2. 图像锐化增强(使用卷积核):
```python
import cv2
import numpy as np
def sharpen_image(image):
kernel = np.array([[-1,-1,-1], [-1,9,-1], [-1,-1,-1]])
图像增强算法代码python翻转 放缩
图像增强算法是指通过对图像进行一系列的处理,使得图像在视觉上更加清晰、鲜明、易于识别。其中包括很多种算法,比如直方图均衡化、对比度增强、锐化等等。这些算法都可以通过Python代码实现。
翻转和放缩是图像增强中的两个常用操作。下面是Python代码实现:
1. 翻转
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 水平翻转
h_flip = cv2.flip(img, 1)
# 垂直翻转
v_flip = cv2.flip(img, 0)
# 对角线翻转
d_flip = cv2.flip(img, -1)
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Horizontal Flip', h_flip)
cv2.imshow('Vertical Flip', v_flip)
cv2.imshow('Diagonal Flip', d_flip)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. 放缩
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 缩小图像
smaller = cv2.resize(img, (0, 0), fx=0.5, fy=0.5)
# 放大图像
larger = cv2.resize(img, (0, 0), fx=1.5, fy=1.5)
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Smaller Image', smaller)
cv2.imshow('Larger Image', larger)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
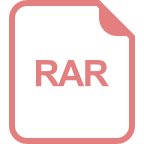
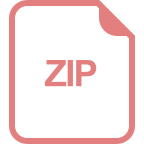
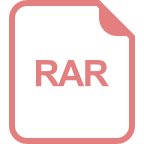












