__add__(self, other)怎么使用
时间: 2023-10-28 09:06:16 浏览: 119
`__add__(self, other)`是Python中定义类的特殊方法之一,用于重载`+`运算符。它允许你在自定义类中使用`+`运算符进行自定义的操作。
例如,假设你有一个名为`Person`的类,其中包含`name`和`age`属性。你可以使用`__add__`方法,使得两个`Person`对象相加时,返回他们年龄的和。
示例代码如下:
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __add__(self, other):
return self.age + other.age
person1 = Person("Alice", 25)
person2 = Person("Bob", 30)
total_age = person1 + person2 # 此处会调用 person1.__add__(person2)
print(total_age) # 输出 55
```
在上面的代码中,我们定义了一个`Person`类,并在`__add__`方法中重载了`+`运算符。当我们将两个`Person`对象相加时,会调用该方法并返回两个人的年龄之和。
需要注意的是,`__add__`方法必须返回一个值,而且该值可以是任意类型。在上面的例子中,我们返回了一个整数,但你也可以返回一个新的`Person`对象或其他类型的对象。
相关问题
class Point: def __init__(self, x, y): self.__x = x self.__y = y def __add__(self, other): x = self.__x + other.__x y = self.__y + other.__y return Point(x, y) def __sub__(self, other): x = self.__x - other.__x y = self.__y - other.__y return Point(x, y) def __mul__(self, a): x = self.__x * a y = self.__y * a return Point(x, y) def __truediv__(self, a): x = self.__x / a y = self.__y / a return Point(x, y) r1 = Point(10, 20) r2 = Point(5, 5) print(r1+r2) print(r1-r2) print(r1*2) print(r1/2)代码改进,输出向量坐标
为了输出向量坐标,我们可以在 Point 类中添加一个方法来返回坐标的元组,如下所示:
```python
class Point:
def __init__(self, x, y):
self.__x = x
self.__y = y
def __add__(self, other):
x = self.__x + other.__x
y = self.__y + other.__y
return Point(x, y)
def __sub__(self, other):
x = self.__x - other.__x
y = self.__y - other.__y
return Point(x, y)
def __mul__(self, a):
x = self.__x * a
y = self.__y * a
return Point(x, y)
def __truediv__(self, a):
x = self.__x / a
y = self.__y / a
return Point(x, y)
def get_coordinates(self):
return (self.__x, self.__y)
```
然后我们就可以使用这个方法来输出向量坐标了,示例如下:
```python
r1 = Point(10, 20)
r2 = Point(5, 5)
print(r1+r2.get_coordinates()) # 输出 (15, 25)
print(r1-r2.get_coordinates()) # 输出 (5, 15)
print(r1*2).get_coordinates()) # 输出 (20, 40)
print(r1/2).get_coordinates()) # 输出 (5.0, 10.0)
```
class Point: # 构造方法,初始化,定义向量坐标 def __init__(self, x, y): self.__x = x self.__y = y # 向量加法,对应分量相加,返回新向量 def __add__(self, other): x = self.__x + other.__x y = self.__y + other.__y return Point(x, y) # 向量减法,对应分量相减,返回新向量 def __sub__(self, other): x = self.__x - other.__x y = self.__y - other.__y return Point(x, y) # 向量乘法,各分量乘以同一个数字,返回新向量 def __mul__(self, a): x = self.__x * a y = self.__y * a return Point(x, y) def __truediv__(self, a): x = self.__x / a y = self.__y / a return Point(x, y) # 若没有这串代码输出的是对象的地址,而不是向量坐标 def show(self): return(self.__x, self.__y) r1 = Point(10, 20) r2 = Point(5, 5) print(r1+r2.show()) print(r1-r2.show()) print((r1*2).show()) print((r1/2).show())错误并改正
错误:在向量加法、向量减法、向量乘法和向量除法的运算中,调用了另一个向量对象的show()方法,导致输出结果不是向量坐标。
改正:在向量加法、向量减法、向量乘法和向量除法的运算中,直接使用另一个向量对象的坐标进行运算即可。
修改后的代码如下:
```python
class Point:
def __init__(self, x, y):
self.__x = x
self.__y = y
def __add__(self, other):
x = self.__x + other.__x
y = self.__y + other.__y
return Point(x, y)
def __sub__(self, other):
x = self.__x - other.__x
y = self.__y - other.__y
return Point(x, y)
def __mul__(self, a):
x = self.__x * a
y = self.__y * a
return Point(x, y)
def __truediv__(self, a):
x = self.__x / a
y = self.__y / a
return Point(x, y)
def show(self):
return (self.__x, self.__y)
r1 = Point(10, 20)
r2 = Point(5, 5)
print((r1+r2).show())
print((r1-r2).show())
print((r1*2).show())
print((r1/2).show())
```
阅读全文
相关推荐
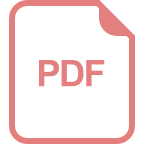














