java使用mybatis写一个具有两个根据条件查询功能的项目要求具体代码实现
时间: 2024-02-21 10:57:01 浏览: 53
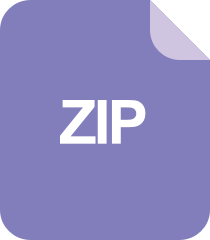
multipleselect:java结合mybatis-plus实现非手写sql多表查询

好的,针对您的问题,我可以给您提供一个简单的示例代码:
首先,我们需要定义两个mapper.xml文件,分别用于实现两个根据条件查询的功能。假设我们需要查询学生信息,其中一个查询功能是根据学生姓名查询,另一个查询功能是根据学生年龄查询。那么我们的mapper.xml文件可以这样定义:
1. 根据学生姓名查询的mapper.xml文件
```xml
<!-- StudentMapper.xml -->
<mapper namespace="com.example.mapper.StudentMapper">
<!-- 根据学生姓名查询 -->
<select id="findByName" resultType="com.example.model.Student">
SELECT * FROM student WHERE name = #{name}
</select>
</mapper>
```
2. 根据学生年龄查询的mapper.xml文件
```xml
<!-- StudentMapper.xml -->
<mapper namespace="com.example.mapper.StudentMapper">
<!-- 根据学生年龄查询 -->
<select id="findByAge" resultType="com.example.model.Student">
SELECT * FROM student WHERE age = #{age}
</select>
</mapper>
```
然后,我们需要定义一个对应的StudentMapper接口,并在接口中定义两个方法,分别对应上面两个mapper.xml文件中的查询功能。代码如下:
```java
// StudentMapper.java
package com.example.mapper;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import com.example.model.Student;
@Mapper
public interface StudentMapper {
// 根据学生姓名查询
List<Student> findByName(@Param("name") String name);
// 根据学生年龄查询
List<Student> findByAge(@Param("age") int age);
}
```
接着,我们需要定义一个StudentService服务类,用于调用StudentMapper接口中的方法,并将查询结果返回给调用方。代码如下:
```java
// StudentService.java
package com.example.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.mapper.StudentMapper;
import com.example.model.Student;
@Service
public class StudentService {
@Autowired
private StudentMapper studentMapper;
// 根据学生姓名查询
public List<Student> findByName(String name) {
return studentMapper.findByName(name);
}
// 根据学生年龄查询
public List<Student> findByAge(int age) {
return studentMapper.findByAge(age);
}
}
```
最后,我们可以在Controller中调用StudentService中的查询方法,并将查询结果返回给前端页面。代码如下:
```java
// StudentController.java
package com.example.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.example.model.Student;
import com.example.service.StudentService;
@Controller
public class StudentController {
@Autowired
private StudentService studentService;
// 根据学生姓名查询
@GetMapping("/findByName")
public String findByName(@RequestParam("name") String name, Model model) {
List<Student> students = studentService.findByName(name);
model.addAttribute("students", students);
return "studentList";
}
// 根据学生年龄查询
@GetMapping("/findByAge")
public String findByAge(@RequestParam("age") int age, Model model) {
List<Student> students = studentService.findByAge(age);
model.addAttribute("students", students);
return "studentList";
}
}
```
以上就是一个简单的使用MyBatis实现两个根据条件查询功能的示例代码。当然,具体实现方式可能会因为业务需求而有所不同,以上示例代码仅供参考。
阅读全文
相关推荐
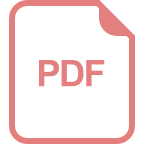
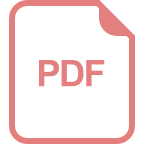















