写Nodejs脚本,遍历文件夹与子文件夹,找到压缩文件并解压,并将压缩文件删除
时间: 2024-01-10 08:03:17 浏览: 95
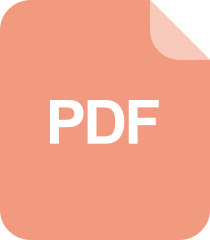
nodejs实现遍历文件夹并统计文件大小
好的,可以使用Node.js自带的`fs`模块和第三方的`adm-zip`模块来实现遍历文件夹、解压和删除压缩文件的操作。具体代码如下:
```javascript
const fs = require('fs');
const path = require('path');
const AdmZip = require('adm-zip');
function unzipAndDeleteZipFiles(dirPath) {
fs.readdir(dirPath, (err, files) => {
if (err) {
console.error(`Failed to read directory ${dirPath}: ${err}`);
return;
}
// 遍历文件夹
files.forEach(file => {
const filePath = path.join(dirPath, file);
// 判断是否为压缩文件
if (fs.statSync(filePath).isFile() && path.extname(file) === '.zip') {
console.log(`Unzipping file: ${filePath}`);
// 解压文件
const zip = new AdmZip(filePath);
zip.extractAllTo(dirPath, true);
// 删除压缩文件
fs.unlinkSync(filePath);
console.log(`Deleted file: ${filePath}`);
} else if (fs.statSync(filePath).isDirectory()) {
// 如果是文件夹,则递归处理子文件夹
unzipAndDeleteZipFiles(filePath);
}
});
});
}
// 测试
unzipAndDeleteZipFiles('/path/to/directory');
```
在上面的代码中,`unzipAndDeleteZipFiles`函数接收一个文件夹路径作为参数,首先读取该文件夹下的所有文件和子文件夹,然后遍历每个文件,如果是压缩文件(这里假设是`.zip`文件),则使用`adm-zip`模块解压该文件到指定文件夹,并删除该压缩文件;如果是文件夹,则递归调用`unzipAndDeleteZipFiles`函数处理子文件夹。
你可以将上面的代码保存为一个`.js`文件,然后在命令行中运行`node`命令来执行该文件,比如:
```
node unzip.js
```
注意要替换`/path/to/directory`为你要遍历的文件夹路径。
阅读全文
相关推荐
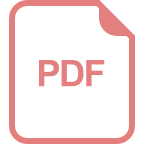















