写nodejs代码,遍历文件夹和子文件夹,如果rar或者zip,7z类的压缩包,需要解压密码,就删掉压缩包
时间: 2024-03-28 21:42:39 浏览: 77
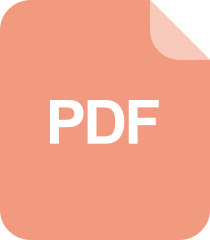
NodeJS 将文件夹按照存放路径变成一个对应的JSON的方法
好的,以下是可以用于遍历文件夹和子文件夹,检查并解压缩压缩文件的Node.js代码:
```javascript
const fs = require('fs');
const path = require('path');
const unzipper = require('unzipper');
const Seven = require('node-7z');
const rootFolder = 'your/root/folder/path';
const password = 'your-password';
fs.readdir(rootFolder, (err, files) => {
if (err) throw err;
files.forEach(file => {
const fileFullPath = path.join(rootFolder, file);
fs.stat(fileFullPath, (err, stats) => {
if (err) throw err;
if (stats.isDirectory()) {
// 如果是文件夹,递归遍历子文件夹
recurseFolder(fileFullPath);
} else {
// 如果是文件,检查是否为压缩文件
const ext = path.extname(file);
if (['.zip', '.rar', '.7z'].includes(ext)) {
console.log(`Found compressed file: ${file}`);
// 解压缩文件
const outputFolder = path.dirname(fileFullPath);
const unzip = ext === '.7z' ?
new Seven().extractFull(fileFullPath, outputFolder, { $: password }) :
fs.createReadStream(fileFullPath).pipe(unzipper.Extract({ path: outputFolder }));
// 监听解压缩事件
unzip.on('error', err => {
console.error(`Failed to extract ${file}: ${err}`);
});
unzip.on('close', () => {
console.log(`Extracted ${file} successfully`);
// 删除压缩文件
fs.unlink(fileFullPath, err => {
if (err) console.error(`Failed to delete ${file}: ${err}`);
else console.log(`Deleted ${file}`);
});
});
}
}
});
});
});
function recurseFolder(folderPath) {
fs.readdir(folderPath, (err, files) => {
if (err) throw err;
files.forEach(file => {
const fileFullPath = path.join(folderPath, file);
fs.stat(fileFullPath, (err, stats) => {
if (err) throw err;
if (stats.isDirectory()) {
// 如果是文件夹,递归遍历子文件夹
recurseFolder(fileFullPath);
} else {
// 如果是文件,检查是否为压缩文件
const ext = path.extname(file);
if (['.zip', '.rar', '.7z'].includes(ext)) {
console.log(`Found compressed file: ${file}`);
// 解压缩文件
const outputFolder = path.dirname(fileFullPath);
const unzip = ext === '.7z' ?
new Seven().extractFull(fileFullPath, outputFolder, { $: password }) :
fs.createReadStream(fileFullPath).pipe(unzipper.Extract({ path: outputFolder }));
// 监听解压缩事件
unzip.on('error', err => {
console.error(`Failed to extract ${file}: ${err}`);
});
unzip.on('close', () => {
console.log(`Extracted ${file} successfully`);
// 删除压缩文件
fs.unlink(fileFullPath, err => {
if (err) console.error(`Failed to delete ${file}: ${err}`);
else console.log(`Deleted ${file}`);
});
});
}
}
});
});
});
}
```
该代码使用了Node.js自带的fs模块来遍历文件夹和子文件夹,并使用了第三方库unzipper来解压缩zip文件,使用了第三方库node-7z来解压缩rar和7z文件。在解压缩文件时,如果需要密码,可以将密码作为参数传递给node-7z库的extractFull()方法。解压缩后,代码会删除原始的压缩文件。
阅读全文
相关推荐
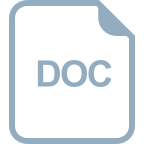
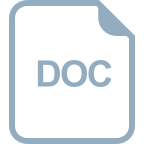


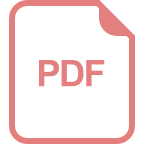
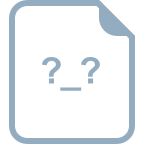
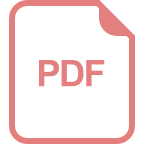







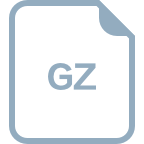
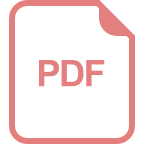