写nodejs代码,遍历文件夹和子文件夹,如果rar或者zip,7z类的压缩包,如果压缩包需要密码,就删掉压缩包。解压成功后也删除掉压缩包
时间: 2024-03-28 21:42:41 浏览: 112
好的,我可以为您提供一个基于Node.js的解决方案。请注意,这个程序将会删除您指定文件夹中的压缩文件,包括需要密码的压缩文件,请谨慎执行。
```
const fs = require('fs');
const path = require('path');
const unzipper = require('unzipper'); // 需要安装 unzipper 模块
const targetDir = '/path/to/your/directory'; // 需要遍历的文件夹路径
function deleteFile(filePath) {
fs.unlinkSync(filePath);
console.log(`Deleted file: ${filePath}`);
}
function unzipFile(filePath, password) {
fs.createReadStream(filePath)
.pipe(unzipper.Extract({ path: path.dirname(filePath), password: password }))
.on('finish', () => {
deleteFile(filePath); // 解压成功后删除压缩文件
});
}
function traverseDir(dirPath) {
const files = fs.readdirSync(dirPath);
files.forEach(file => {
const filePath = path.join(dirPath, file);
const fileStat = fs.statSync(filePath);
if (fileStat.isDirectory()) {
traverseDir(filePath);
} else {
const extName = path.extname(filePath).toLowerCase();
if (['.zip', '.rar', '.7z'].includes(extName)) {
if (extName === '.zip') {
unzipFile(filePath, ''); // 如果不需要密码,将 password 参数设为空字符串
} else {
deleteFile(filePath); // 其他类型的压缩文件直接删除
}
}
}
});
}
traverseDir(targetDir);
```
请将代码中的 `/path/to/your/directory` 替换成您想要遍历的文件夹路径,并且确保您已经安装了 `unzipper` 模块。
阅读全文
相关推荐
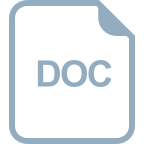
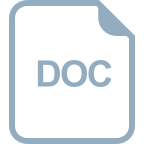
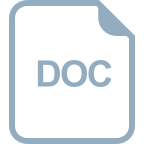

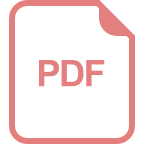

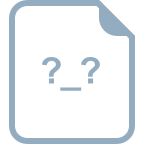
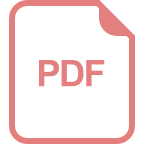







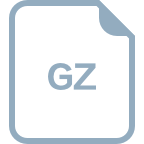
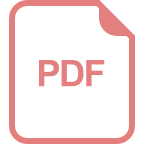