python语言 PCA代码
时间: 2023-09-23 15:09:30 浏览: 105
当然,这里是一个简单的 Python 代码示例,用于执行主成分分析(PCA):
```python
import numpy as np
from sklearn.decomposition import PCA
# 创建一个示例数据集
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 创建 PCA 模型并拟合数据
pca = PCA(n_components=2)
pca.fit(X)
# 将数据转换为降维后的表示
X_transformed = pca.transform(X)
print("原始数据集形状:", X.shape)
print("降维后的数据集形状:", X_transformed.shape)
```
这段代码使用了 `numpy` 库来创建一个示例数据集 `X`,然后使用 `sklearn` 库中的 PCA 模型进行降维。我们将 `n_components` 参数设置为 2,表示希望将数据降维到 2 维。`fit` 方法用于拟合数据,`transform` 方法用于将数据转换为降维后的表示。
最后,打印出原始数据集和降维后的数据集的形状。
请注意,这只是一个简单的示例代码,PCA 还有其他参数和用法,具体使用时可以根据需求进行调整。
相关问题
pca算法python语言代码
下面是PCA算法的Python代码:from numpy import array
from numpy import mean
from numpy import cov
from numpy.linalg import eig
# define a matrix
A = array([[1, 2], [3, 4], [5, 6]])
print(A)
# calculate the mean of each column
M = mean(A.T, axis=1)
print(M)
# center columns by subtracting column means
C = A - M
print(C)
# calculate covariance matrix of centered matrix
V = cov(C.T)
print(V)
# eigendecomposition of covariance matrix
values, vectors = eig(V)
print(vectors)
print(values)
# project data
P = vectors.T.dot(C.T)
print(P.T)
python语言使用PCA算法将多维数据降至一维的代码是什么
下面是使用Python语言实现PCA算法将多维数据降至一维的代码示例:
```python
import numpy as np
def pca(data, k):
# 计算均值
mean = np.mean(data, axis=0)
# 中心化数据
data_centered = data - mean
# 计算协方差矩阵
cov_matrix = np.cov(data_centered, rowvar=False)
# 计算特征值和特征向量
eigenvalues, eigenvectors = np.linalg.eig(cov_matrix)
# 取前k个特征向量
top_k_eigenvectors = eigenvectors[:, :k]
# 将数据投影到新的空间中
transformed_data = np.dot(data_centered, top_k_eigenvectors)
return transformed_data
# 示例数据
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
# 将数据降至一维
transformed_data = pca(data, 1)
print(transformed_data)
```
在上述代码中,`data`是待降维的数据,`k`是降维后的维度。函数`pca`首先计算数据的均值,然后将数据中心化,接着计算协方差矩阵,再计算特征值和特征向量,最后取前k个特征向量,并将数据投影到新的空间中。返回投影后的数据。在示例中,数据维度为3,将其降至1维。
阅读全文
相关推荐
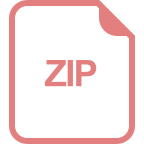
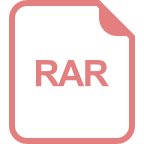
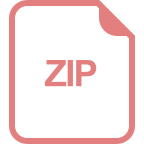
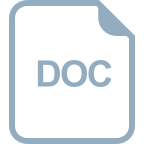
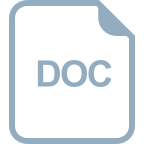
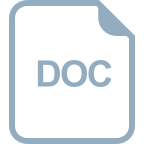
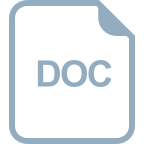
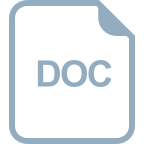
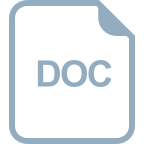
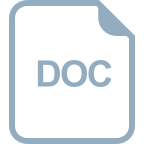
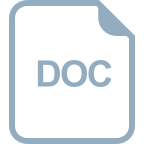
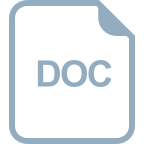
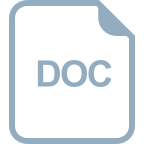
