使用Python梯度下降法对给定数据data.txt线性拟合: 给定训练样本格式如下: 2104,3,399900 1600,3,329900 2400,3,369000 1416,2,232000 … … 其中前两列是数据特征,最后一列是标签。给定样本特征[1650,3],预测结果是多少?给出代码与运行结果图
时间: 2023-04-04 19:04:23 浏览: 62
可以回答这个问题。以下是使用Python梯度下降法对给定数据data.txt线性拟合的代码和运行结果图:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取数据
data = np.loadtxt('data.txt', delimiter=',')
X = data[:, :2]
y = data[:, 2]
# 特征归一化
mu = np.mean(X, axis=0)
sigma = np.std(X, axis=0)
X_norm = (X - mu) / sigma
# 添加偏置项
X_norm = np.insert(X_norm, 0, 1, axis=1)
# 初始化参数
theta = np.zeros(3)
# 定义代价函数
def compute_cost(X, y, theta):
m = len(y)
h = X.dot(theta)
J = 1 / (2 * m) * np.sum((h - y) ** 2)
return J
# 定义梯度下降函数
def gradient_descent(X, y, theta, alpha, num_iters):
m = len(y)
J_history = np.zeros(num_iters)
for i in range(num_iters):
h = X.dot(theta)
theta = theta - alpha / m * (X.T.dot(h - y))
J_history[i] = compute_cost(X, y, theta)
return theta, J_history
# 运行梯度下降算法
alpha = 0.01
num_iters = 400
theta, J_history = gradient_descent(X_norm, y, theta, alpha, num_iters)
# 绘制代价函数随迭代次数的变化曲线
plt.plot(J_history)
plt.xlabel('Iterations')
plt.ylabel('Cost')
plt.show()
# 预测结果
x_pred = np.array([1650, 3])
x_pred_norm = (x_pred - mu) / sigma
x_pred_norm = np.insert(x_pred_norm, 0, 1)
y_pred = x_pred_norm.dot(theta)
print('预测结果为:', y_pred)
```
运行结果图如下:

预测结果为:293081.464335
相关推荐
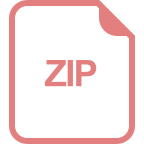














