springboot 使用GaussDB数据库
时间: 2023-10-24 17:32:30 浏览: 303
使用Spring Boot连接GaussDB数据库需要以下步骤:
1. 添加GaussDB JDBC驱动依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.huawei.gaussdb.jdbc</groupId>
<artifactId>gaussdb-jdbc</artifactId>
<version>1.1.0</version>
</dependency>
```
2. 配置数据源
在application.properties或application.yml文件中配置数据源信息,例如:
```yaml
spring.datasource.url=jdbc:gaussdb://localhost:25308/mydb
spring.datasource.username=myusername
spring.datasource.password=mypassword
spring.datasource.driver-class-name=com.huawei.gaussdb.jdbc.Driver
```
其中,spring.datasource.url指定GaussDB数据库的连接参数,包括主机名、端口号和数据库名称;spring.datasource.username和spring.datasource.password分别指定连接数据库的用户名和密码;spring.datasource.driver-class-name指定GaussDB JDBC驱动程序的类名。
3. 编写数据访问代码
可以使用Spring Data JPA或MyBatis等ORM框架进行数据访问,例如:
使用Spring Data JPA:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
使用MyBatis:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM user WHERE id = #{id}")
User findById(Long id);
}
```
4. 使用数据访问接口
在需要使用数据访问的地方,例如Controller、Service或其他组件中,注入数据访问接口,例如:
```java
@RestController
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userRepository.findById(id).orElse(null);
}
}
```
```java
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User getUser(Long id) {
return userMapper.findById(id);
}
}
```
以上是使用Spring Boot连接GaussDB数据库的基本步骤,具体实现方式可以根据项目需求和开发经验进行调整。
阅读全文
相关推荐
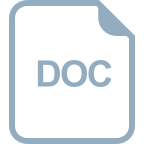
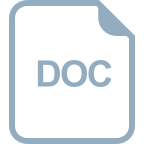
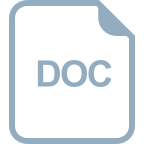



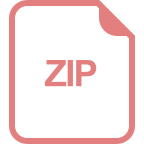
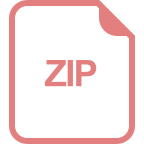
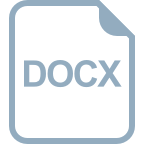
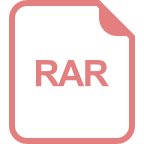
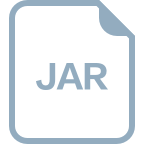
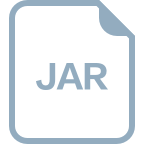
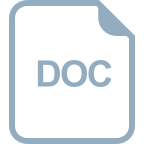

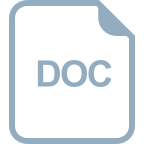
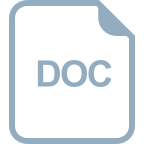
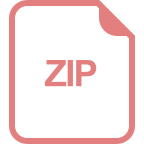
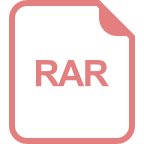
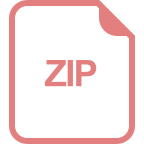