Define a Pet class that stores the pet’s name, age, and weight. Add appropriate constructors, accessor, and mutator functions. Also define a function named getLifespan that returns a string with the value “unknown lifespan.” Next, define a Dog class that is derived from Pet. The Dog class should have a private member variable named breed that stores the breed of the dog. Add mutator and accessor functions for the breed variable and appropriate constructors. Redefine the getLifespan function to return "Approximately 7 years" if the dog’s weight is over 100 pounds and "Approximately 13 years" if the dog’s weight is under 100 pounds. Next, define a Rock class that is derived from Pet. Redefine the getLifespan function to return “Thousands of years”. Finally, write a test program that creates instances of pet rocks and pet dogs that exercise the inherited and redefined functions.
时间: 2024-03-24 11:38:47 浏览: 74
Sure, here's an example implementation in Python:
```python
class Pet:
def __init__(self, name, age, weight):
self.name = name
self.age = age
self.weight = weight
def get_name(self):
return self.name
def get_age(self):
return self.age
def get_weight(self):
return self.weight
def set_name(self, name):
self.name = name
def set_age(self, age):
self.age = age
def set_weight(self, weight):
self.weight = weight
def get_lifespan(self):
return "unknown lifespan"
class Dog(Pet):
def __init__(self, name, age, weight, breed):
super().__init__(name, age, weight)
self.breed = breed
def get_breed(self):
return self.breed
def set_breed(self, breed):
self.breed = breed
def get_lifespan(self):
if self.weight > 100:
return "Approximately 7 years"
else:
return "Approximately 13 years"
class Rock(Pet):
def get_lifespan(self):
return "Thousands of years"
# Test program
dog1 = Dog("Fido", 3, 75, "Labrador")
dog2 = Dog("Rex", 5, 120, "Great Dane")
rock1 = Rock("Rocky", 1000000, 10)
print(dog1.get_name(), dog1.get_lifespan()) # Fido Approximately 13 years
print(dog2.get_name(), dog2.get_lifespan()) # Rex Approximately 7 years
print(rock1.get_name(), rock1.get_lifespan()) # Rocky Thousands of years
```
In this implementation, the `Pet` class stores the pet's name, age, and weight, and defines getter and setter methods for each attribute. It also defines a `get_lifespan` method that returns "unknown lifespan" by default.
The `Dog` class is derived from `Pet` and adds a `breed` attribute with getter and setter methods. It also redefines the `get_lifespan` method to return different values depending on the dog's weight.
The `Rock` class is also derived from `Pet` and redefines the `get_lifespan` method to return a fixed value.
Finally, the test program creates instances of `Dog` and `Rock` and calls their methods to verify that the inheritance and redefined methods work correctly.
阅读全文
相关推荐
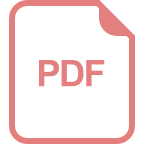
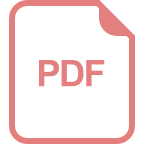
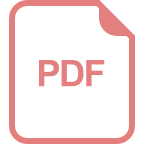





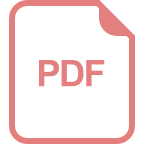
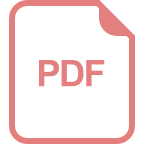
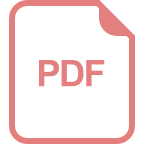
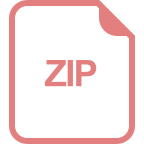
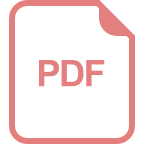
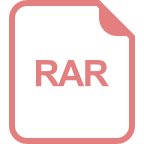
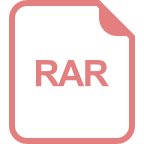
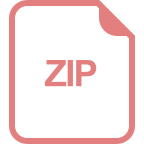
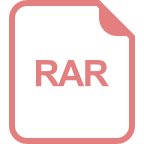
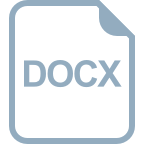