C# 判断list时间是否有重叠,如果有重叠取重叠时间优先级最高的数据
时间: 2024-03-27 10:37:07 浏览: 26
您可以使用C#中的LINQ语言来实现判断List中时间是否有重叠,并取重叠时间优先级最高的数据。以下是一个示例代码,其中假设您有一个包含时间段和优先级的列表,需要判断时间段是否有重叠并取出重叠时间优先级最高的数据。
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
public class Program {
public static void Main() {
// 假设您有一个包含时间段和优先级的列表
var timeIntervals = new List<(DateTime start, DateTime end, int priority)> {
(new DateTime(2021, 10, 1, 9, 0, 0), new DateTime(2021, 10, 1, 10, 0, 0), 2),
(new DateTime(2021, 10, 1, 10, 0, 0), new DateTime(2021, 10, 1, 11, 0, 0), 1),
(new DateTime(2021, 10, 1, 11, 0, 0), new DateTime(2021, 10, 1, 12, 0, 0), 3),
(new DateTime(2021, 10, 1, 12, 0, 0), new DateTime(2021, 10, 1, 13, 0, 0), 2),
(new DateTime(2021, 10, 1, 13, 0, 0), new DateTime(2021, 10, 1, 14, 0, 0), 1)
};
// 首先按照优先级进行排序
var sortedIntervals = timeIntervals.OrderByDescending(ti => ti.priority);
// 然后使用LINQ的Any方法来判断是否有重叠的时间段
var overlappingIntervals = sortedIntervals.Where(ti1 =>
sortedIntervals.Any(ti2 =>
ti2.start < ti1.end && ti2.end > ti1.start && ti1 != ti2
)
);
// 如果有重叠的时间段,则取优先级最高的时间段
if (overlappingIntervals.Any()) {
var highestPriorityInterval = overlappingIntervals.First();
Console.WriteLine($"Overlapping interval: {highestPriorityInterval.start} - {highestPriorityInterval.end} (priority: {highestPriorityInterval.priority})");
} else {
Console.WriteLine("No overlapping intervals found.");
}
}
}
```
在这个示例中,我们首先按照优先级进行排序,将优先级较高的时间段排在前面。然后,我们使用LINQ的Any方法来判断是否有重叠的时间段,其中我们使用两个时间段的开始时间和结束时间进行比较。如果有重叠的时间段,则我们使用LINQ的First方法取优先级最高的时间段,并输出到控制台。如果没有重叠的时间段,则输出"No overlapping intervals found."。
相关推荐
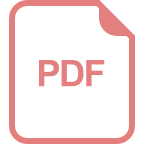














