C++实现密文输入案例
时间: 2024-09-10 15:16:22 浏览: 15
在C++中,实现密文输入的一个简单例子通常涉及到用户输入明文,然后对其进行加密后再显示。这里我们可以使用简单的替换密码或者更复杂的加密算法如Caesar Cipher(凯撒密码)。以下是使用Caesar Cipher的一个简要示例:
```cpp
#include <iostream>
#include <string>
// Caesar Cipher function with shift parameter
std::string caesarCipher(const std::string& plaintext, int shift) {
std::string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) { // Check if character is a letter
char shifted = static_cast<char>((c + shift - 'A') % 26 + 'A'); // Shift and wrap around the alphabet
ciphertext += shifted;
} else {
ciphertext += c; // Leave non-alphabetic characters unchanged
}
}
return ciphertext;
}
int main() {
std::string message;
std::cout << "请输入明文: ";
getline(std::cin, message); // Read input without newline
int shift;
std::cout << "请输入偏移量: ";
std::cin >> shift;
std::string encrypted = caesarCipher(message, shift);
std::cout << "加密后的密文是: " << encrypted << std::endl;
return 0;
}
```
在这个程序中,用户会被提示输入明文和一个偏移值,然后`caesarCipher`函数会将每个字母按照指定的偏移量进行加密。注意这只是一个基础的加密示例,实际应用中可能需要考虑更多的细节,比如处理大小写字母、特殊字符以及输入验证。
相关推荐
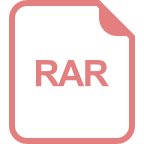
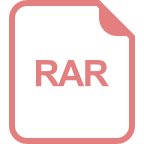
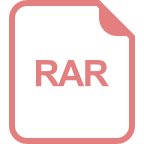














