c++实现凯撒加解密算法
时间: 2024-05-12 16:11:25 浏览: 91
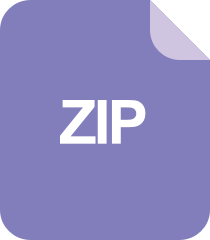
凯撒密码C++的实现
凯撒加解密算法是一种古老的密码学方法,它是一种简单的替换密码。这个算法的基本思想就是将明文中的每个字符按照一定的规则进行替换,从而得到密文。在加密时,将明文中的每个字符向后移动n个位置,而在解密时,将密文中的每个字符向前移动n个位置。
在C++中实现凯撒加解密算法非常简单,只需要将明文和密文中的每个字符进行ASCII码的加减操作即可。
以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
string encrypt(string plaintext, int shift) {
string ciphertext = "";
for (int i = 0; i < plaintext.length(); i++) {
char c = plaintext[i];
if (isalpha(c)) {
c = toupper(c);
c = ((c - 65 + shift) % 26) + 65;
}
ciphertext += c;
}
return ciphertext;
}
string decrypt(string ciphertext, int shift) {
string plaintext = "";
for (int i = 0; i < ciphertext.length(); i++) {
char c = ciphertext[i];
if (isalpha(c)) {
c = toupper(c);
c = ((c - 65 - shift + 26) % 26) + 65;
}
plaintext += c;
}
return plaintext;
}
int main() {
string plaintext = "HELLO WORLD";
int shift = 3;
string ciphertext = encrypt(plaintext, shift);
cout << "Ciphertext: " << ciphertext << endl;
string decryptedtext = decrypt(ciphertext, shift);
cout << "Decryptedtext: " << decryptedtext << endl;
return 0;
}
```
阅读全文
相关推荐
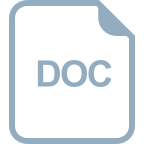
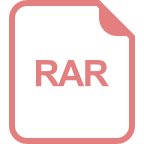
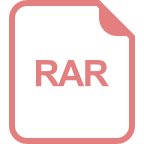
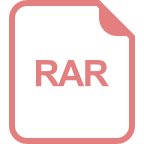
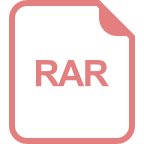
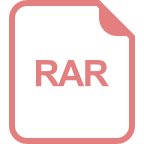
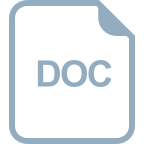
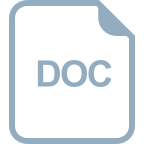
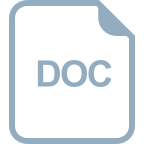
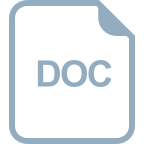
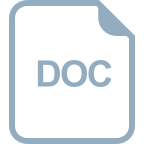



