c++实现导入文本后加密和解密算法
时间: 2024-09-09 16:08:11 浏览: 18
在C++中实现文本的导入、加密和解密通常涉及以下步骤:
1. **文本导入**:首先,需要将文本文件读入到内存中。这通常通过使用标准库中的`ifstream`来完成。例如,可以使用`std::ifstream`打开文件,并使用`std::getline`函数逐行或逐字符读取文件内容。
2. **加密算法**:接着,使用选定的加密算法对读取的文本进行加密。常见的加密算法有凯撒密码、DES、AES等。以简单的凯撒密码为例,它通过将字母表中的每个字母移动固定数目的位置来进行加密。例如,移动3个位置的凯撒密码会将'A'转换为'D','B'转换为'E',依此类推。
3. **解密算法**:解密过程通常是加密过程的逆过程。对于凯撒密码,解密同样是将每个字母向后移动固定数目的位置。如果加密时向右移动了3个位置,解密时则需要向左移动3个位置。
以下是一个简单的凯撒密码加密和解密的C++示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
// 凯撒密码加密函数
std::string caesarEncrypt(const std::string &text, int shift) {
std::string encryptedText = text;
for (char &c : encryptedText) {
if (isalpha(c)) {
char base = isupper(c) ? 'A' : 'a';
c = static_cast<char>((c - base + shift) % 26 + base);
}
}
return encryptedText;
}
// 凯撒密码解密函数
std::string caesarDecrypt(const std::string &text, int shift) {
// 解密的位移是加密位移的负值
return caesarEncrypt(text, -shift);
}
int main() {
std::string filename = "example.txt";
std::ifstream file(filename);
std::string text;
// 读取文件内容
if (file.is_open()) {
std::getline(file, text);
file.close();
} else {
std::cerr << "无法打开文件:" << filename << std::endl;
return 1;
}
// 加密
int shift = 3; // 位移量
std::string encrypted = caesarEncrypt(text, shift);
std::cout << "加密后的文本:" << encrypted << std::endl;
// 解密
std::string decrypted = caesarDecrypt(encrypted, shift);
std::cout << "解密后的文本:" << decrypted << std::endl;
return 0;
}
```
在这个示例中,我们定义了两个函数`caesarEncrypt`和`caesarDecrypt`分别用于加密和解密文本。我们还使用了`std::ifstream`和`std::getline`来从文件中读取文本。
相关推荐
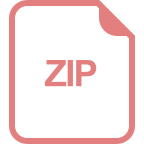
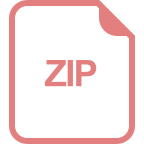
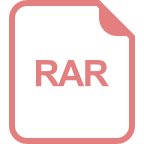














