用c++实现SM4加密算法
时间: 2023-12-13 12:32:14 浏览: 129
以下是使用C++实现SM4加密算法的代码示例:
```c++
#include <iostream>
#include <cstring>
#include <openssl/evp.h>
#include <openssl/err.h>
#include <openssl/sm4.h>
using namespace std;
int main()
{
// 明文和密钥
unsigned char plaintext[] = "Hello, world!";
unsigned char key[] = "1234567890abcdef";
// 初始化SM4加密上下文
SM4_KEY sm4_key;
SM4_set_key(key, &sm4_key);
// 计算加密后的密文长度
int plaintext_len = strlen((char*)plaintext);
int ciphertext_len = plaintext_len + SM4_BLOCK_SIZE - plaintext_len % SM4_BLOCK_SIZE;
// 分配内存
unsigned char* ciphertext = new unsigned char[ciphertext_len]; memset(ciphertext, 0, ciphertext_len);
// 加密
SM4_cbc_encrypt(plaintext, ciphertext, ciphertext_len, &sm4_key, key, SM4_ENCRYPT);
// 输出密文
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 释放内存
delete[] ciphertext;
return 0;
}
```
上述代码使用了OpenSSL库中的SM4加密算法实现了对明文的加密。首先需要初始化SM4加密上下文,然后计算加密后的密文长度,分配内存,最后调用SM4_cbc_encrypt函数进行加密。加密后的密文以16进制形式输出。
阅读全文
相关推荐
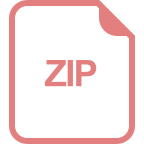

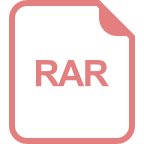
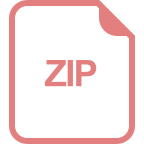
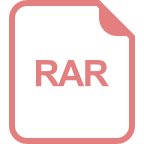
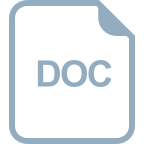
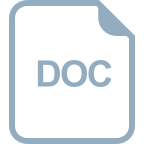







