C++实现字符串加密算法
时间: 2024-09-16 21:01:35 浏览: 10
在C++中,实现字符串加密算法通常涉及到一些常见的加密技术,如替换密码、凯撒密码、简单的位移(比如XOR操作)、哈希函数或者是更复杂的加密标准,如AES、RSA等。这里以简单的凯撒密码为例说明:
凯撒密码是一种替换式加密方法,通过将字母表中的每个字符向后移动固定数量的位置来进行加密。例如,如果偏移量是3,那么"A"会被加密成"D","B"会变成"E",依此类推。
以下是简单的凯撒密码加密算法的C++示例:
```cpp
#include <iostream>
#include <string>
std::string caesarCipher(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否是字母
char encryptedChar = islower(c) ? static_cast<char>(c + shift - 'a') : static_cast<char>(c + shift - 'A');
// 避免超出字母范围
encryptedChar = (encryptedChar > 'z') ? encryptedChar - 26 : encryptedChar;
ciphertext += encryptedChar;
} else {
ciphertext += c; // 非字母字符保持不变
}
}
return ciphertext;
}
int main() {
std::string input = "Hello, World!";
int shift = 3;
std::string encrypted = caesarCipher(input, shift);
std::cout << "Encrypted text: " << encrypted << std::endl;
return 0;
}
```
在这个例子中,`plaintext`是要加密的原始字符串,`shift`是偏移值。注意,这个简单版本的凯撒密码并不适合处理大范围的数据或安全需求高的场景。
相关推荐
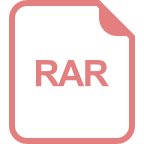
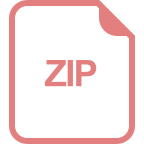
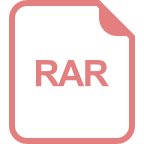














