c++如何将一个字符串加密
时间: 2024-04-28 22:18:46 浏览: 195
C++ 中可以使用加密算法对字符串进行加密。常见的加密算法有对称加密算法和非对称加密算法。
对称加密算法是指加密和解密使用相同的密钥,常见的有DES、AES、RC4等。可以使用库函数或第三方库实现对称加密,比如使用 OpenSSL 库中的 AES 加密算法。下面是一个简单的示例:
```cpp
#include <openssl/aes.h>
#include <string.h>
#include <iostream>
int main() {
std::string plaintext = "hello world";
std::string key = "1234567890123456";
AES_KEY aes_key;
if (AES_set_encrypt_key((unsigned char*)key.c_str(), 128, &aes_key) < 0) {
std::cerr << "AES_set_encrypt_key error" << std::endl;
return -1;
}
unsigned char iv[AES_BLOCK_SIZE] = {0};
std::string ciphertext = plaintext;
AES_cbc_encrypt((unsigned char*)plaintext.c_str(), (unsigned char*)ciphertext.c_str(), plaintext.size(), &aes_key, iv, AES_ENCRYPT);
std::cout << "plaintext: " << plaintext << std::endl;
std::cout << "ciphertext: " << ciphertext << std::endl;
return 0;
}
```
非对称加密算法是指加密和解密使用不同的密钥,常见的有RSA、DSA等。可以使用库函数或第三方库实现非对称加密,比如使用 OpenSSL 库中的 RSA 加密算法。下面是一个简单的示例:
```cpp
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <string.h>
#include <iostream>
int main() {
std::string plaintext = "hello world";
std::string pubkey = "-----BEGIN PUBLIC KEY-----\n\
MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDAG+e+cJgYXmrf3j4Jz6yZi3j\n\
4wEPteL0sXqIcO9S4zV4GFnoCl6F6h9YvyWjV9wN9SIqKt6gMv1BcK3F9y5GJGyP\n\
+Jg2GwLWtS7Jf9yKkH8I6v3mYotTjJZUvWBFi6S9LsQ7OxHfZ8k9ZwWUjH5cK5CM\n\
4SbNQhO5N1Lg7xFW/wIDAQAB\n\
-----END PUBLIC KEY-----";
BIO* bio = BIO_new_mem_buf((void*)pubkey.c_str(), pubkey.size());
if (!bio) {
std::cerr << "BIO_new_mem_buf error" << std::endl;
return -1;
}
RSA* rsa = PEM_read_bio_RSA_PUBKEY(bio, NULL, NULL, NULL);
if (!rsa) {
std::cerr << "PEM_read_bio_RSA_PUBKEY error" << std::endl;
return -1;
}
int rsa_len = RSA_size(rsa);
unsigned char* ciphertext = new unsigned char[rsa_len];
int ret = RSA_public_encrypt(plaintext.size(), (unsigned char*)plaintext.c_str(), ciphertext, rsa, RSA_PKCS1_PADDING);
if (ret < 0) {
std::cerr << "RSA_public_encrypt error" << std::endl;
return -1;
}
std::string ciphertext_str((char*)ciphertext, ret);
std::cout << "plaintext: " << plaintext << std::endl;
std::cout << "ciphertext: " << ciphertext_str << std::endl;
RSA_free(rsa);
BIO_free(bio);
delete[] ciphertext;
return 0;
}
```
阅读全文
相关推荐
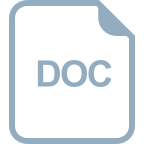
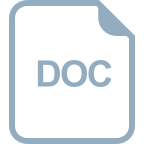
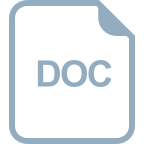
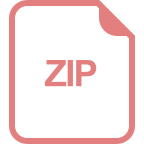

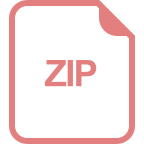
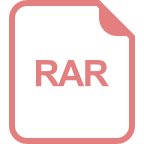
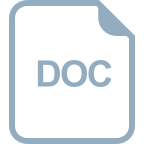
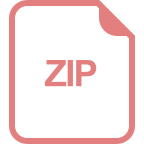
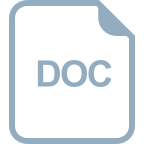
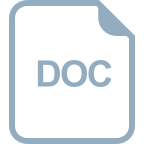
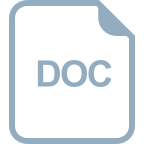
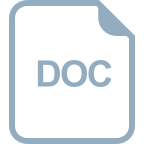





