实现凯撒密码算法的代码用C++语言实现”
时间: 2024-10-13 21:05:42 浏览: 56
凯撒密码是一种简单的替换加密技术,通过将字母表中的每个字符按照固定的偏移量进行位移来加密信息。以下是使用C++实现凯撒密码的一个简单示例:
```cpp
#include <iostream>
#include <cctype>
// 函数用于加密字符串
std::string caesar_cipher_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext = "";
for (char c : plaintext) {
if (isalpha(c)) { // 检查是否为字母
char shifted = static_cast<char>((c + shift - 'A') % 26 + 'A'); // 对大写字母进行加密
if (islower(c))
shifted = static_cast<char>((c + shift - 'a') % 26 + 'a'); // 对小写字母进行加密
ciphertext += shifted;
} else {
ciphertext += c; // 非字母字符保持不变
}
}
return ciphertext;
}
// 函数用于解密字符串
std::string caesar_cipher_decrypt(const std::string& ciphertext, int shift) {
return caesar_cipher_encrypt(ciphertext, -shift);
}
int main() {
std::string message = "Hello, World!";
int shift_amount = 3; // 示例偏移量
std::cout << "Original message: " << message << std::endl;
std::string encrypted = caesar_cipher_encrypt(message, shift_amount);
std::cout << "Encrypted message (shifted by " << shift_amount << "): " << encrypted << std::endl;
std::string decrypted = caesar_cipher_decrypt(encrypted, shift_amount);
std::cout << "Decrypted message: " << decrypted << std::endl;
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`函数负责加密,`caesar_cipher_decrypt`函数负责解密,用户可以自定义偏移量`shift_amount`来改变加密强度。
阅读全文
相关推荐
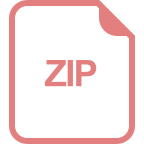
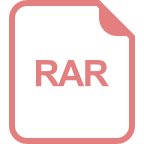
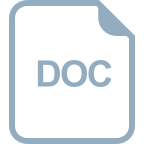


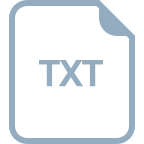
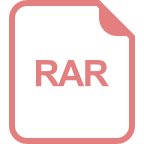
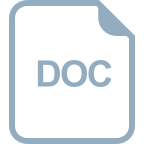
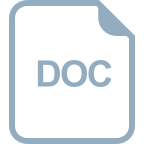
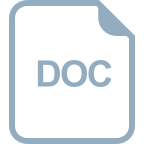
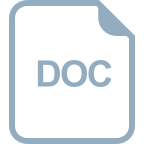
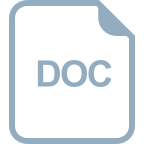
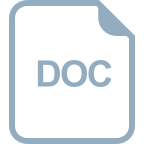


