c语言设计一个算法求串s所含不同字符的总数和每种字符的个数。假设串采用链串存储结构。用相关数据进行测试。
时间: 2024-10-23 07:08:01 浏览: 29
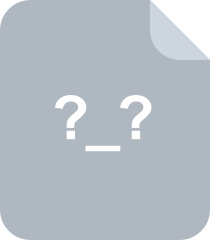
求一个字符串中字母的个数,以及一个字符串中数字的个数.md

在 C 语言中,我们可以使用哈希表(散列表)或者数组配合链表结构来设计一个算法,用于统计字符串 `s` 中的不同字符及其各自的计数。这里我将展示一种基于哈希表的解决方案:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
char data;
struct Node* next;
} ListNode;
// 初始化链表节点
ListNode* createNode(char ch) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = ch;
newNode->next = NULL;
return newNode;
}
// 创建链串
ListNode* createChainString(char* s) {
ListNode* head = NULL;
for (int i = 0; s[i] != '\0'; i++) {
ListNode* newNode = createNode(s[i]);
if (!head) {
head = newNode;
} else {
ListNode* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = newNode;
}
}
return head;
}
// 计算链串中不同字符的数量和计数
void countDistinctChars(ListNode* head) {
int count[256] = {0}; // 用256长度的数组覆盖ASCII码范围
ListNode* current = head;
while (current) {
count[current->data]++;
current = current->next;
}
int distinctCount = 0;
printf("不同字符的数量: ");
for (int i = 0; i < 256; i++) {
if (count[i] > 0) {
printf("%d(%d次), ", count[i], i);
distinctCount++;
}
}
printf("\n总计 %d 个不同字符\n", distinctCount);
free(current); // 清理链表内存
}
int main() {
char str[] = "Hello, World!";
ListNode* chain = createChainString(str);
countDistinctChars(chain);
return 0;
}
```
在这个示例中,我们首先创建一个链串结构表示输入的字符串,然后遍历这个链串,使用一个数组 `count` 来记录每个字符出现的次数。最后,我们计算并打印出不同字符的数量以及它们各自出现的次数。
**相关问题--:**
1. 如果字符串非常大,这种方法的时间复杂度是多少?
2. 这个算法能处理非 ASCII 字符吗?
3. 如何优化这个算法,使得它能在空间上更节省?
阅读全文
相关推荐
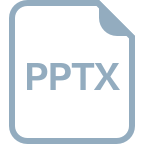
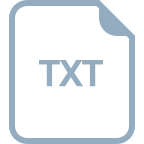
















