C语言利用串的基本操作及匹配算法实现如下功能: 输入两个字符串 s 和 t,统计串 s 中包含串 t 的个数。
时间: 2024-12-13 16:19:14 浏览: 8
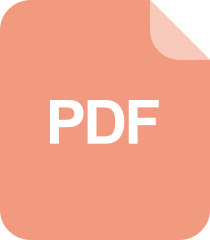
C语言数据结构之模式匹配字符串定位问题

在C语言中,我们可以使用循环和字符串匹配算法(如KMP算法或Boyer-Moore算法)来计算一个字符串s中另一个字符串t出现的次数。下面是一个基于朴素方法(逐字符比较)的简单示例,它的时间复杂度较高,但易于理解。若需要更高效的算法,可以选择KMP或Boyer-Moore等高级算法,但这已经超出了基本C语言教程的范围。
首先,我们定义一个函数来计算字符串中子串的出现次数:
```c
#include <stdio.h>
#include <string.h>
// 函数用于计算字符串s中字符串t出现的次数
int countOccurrences(char *s, char *t) {
int i = 0, j = 0, count = 0;
while (i < strlen(s)) {
if (j == strlen(t)) { // 如果找到一个完整的子串t
count++;
j = 0; // 更新指针
} else if (s[i] == t[j]) { // 字符相等,继续比较下一个
i++;
j++;
} else { // 字符不相等,尝试回溯
if (j > 0) j--;
else i++; // 如果j已经是0了,说明s的前部分不符合t,直接移动到下一位s
}
}
return count;
}
int main() {
char str[100], substr[100];
printf("请输入第一个字符串s: ");
fgets(str, sizeof(str), stdin); // 注意处理换行符
str[strlen(str) - 1] = '\0'; // 去除fgets读取的换行符
printf("请输入子串t: ");
fgets(substr, sizeof(substr), stdin);
substr[strlen(substr) - 1] = '\0';
int occurrences = countOccurrences(str, substr);
printf("字符串s中包含字符串t的次数为: %d\n", occurrences);
return 0;
}
```
阅读全文
相关推荐
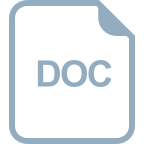
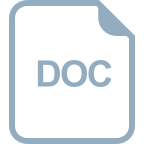
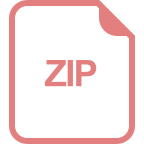
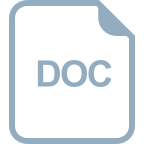
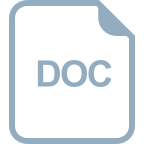
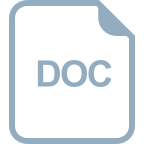
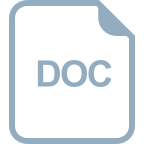
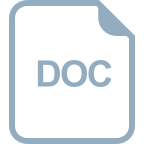
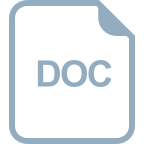
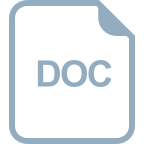
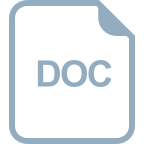
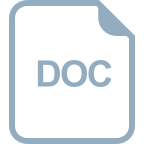




