假设二叉树中的每个结点值为单个字符,采用顺序存储结构存储。设计一个算法,使用C语言求二叉树t中的叶子结点个数
时间: 2024-01-22 20:18:33 浏览: 83
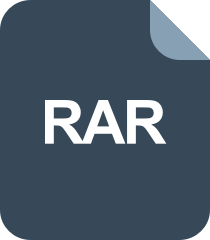
用C语言求二叉树叶子结点

可以使用递归的方式求解二叉树中的叶子结点个数。具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_TREE_SIZE 100
typedef char ElemType;
// 顺序存储结构的二叉树
typedef struct {
ElemType data[MAX_TREE_SIZE]; // 存储结点数据
int n; // 结点个数
} SqBinaryTree;
// 求二叉树中叶子结点的个数
int countLeafNodes(SqBinaryTree t, int root) {
// 如果根结点为空,则叶子结点个数为0
if (root == 0) {
return 0;
}
// 如果根结点没有左右子树,则根结点是叶子结点
else if (t.data[root] != '#' && t.data[root * 2] == '#' && t.data[root * 2 + 1] == '#') {
return 1;
}
// 否则,递归求解左右子树的叶子结点个数
else {
return countLeafNodes(t, root * 2) + countLeafNodes(t, root * 2 + 1);
}
}
int main() {
SqBinaryTree t = {{'#', 'A', 'B', 'C', '#', 'D', '#', '#', 'E', '#', 'F'}, 10};
int root = 1; // 根结点下标为1
printf("叶子结点个数为:%d\n", countLeafNodes(t, root)); // 输出结果为3
return 0;
}
```
注意,上述算法实现中,我们假设二叉树中的空结点用字符`'#'`来表示。同时,我们假设二叉树的根结点下标为1,即`t.data[1]`为根结点的值。
阅读全文
相关推荐










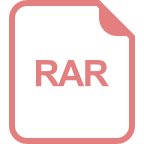
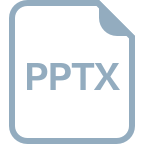
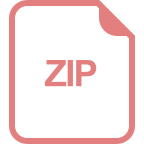
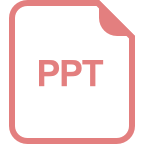
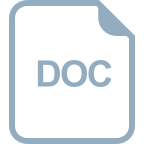
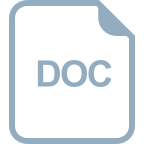