message = sc.nextLine();
时间: 2024-04-09 12:32:14 浏览: 57
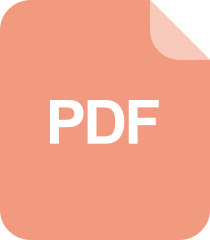
浅析java中next与nextLine用法对比
在您提供的代码片段中,"sc.nextLine()"是用于从输入流中读取下一行文本的代码。通常情况下,"sc"是一个Scanner对象,它可以用于读取来自客户端的输入。
这行代码将阻塞程序的执行,直到从输入流中读取到一行文本为止。一旦读取到文本,它将被存储在名为"message"的变量中,您可以在接下来的代码中使用这个变量来处理接收到的消息。
请注意,这里假设您已经正确地设置了与客户端的连接,并且已经创建了一个Scanner对象"sc"来读取客户端输入。具体的实现方式将取决于您的应用程序和所使用的编程语言。
阅读全文
相关推荐
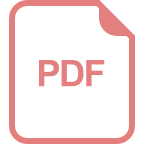
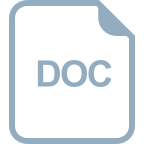




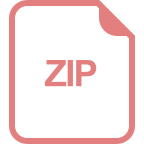
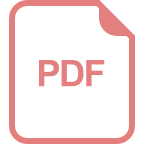
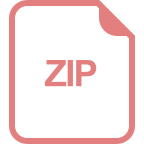
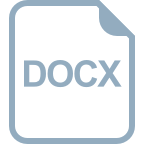
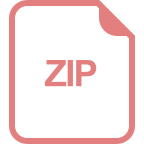
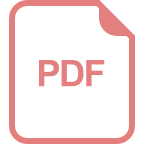