faster rcnn实现有丝分裂细胞目标检测python代码
时间: 2023-09-12 07:10:35 浏览: 53
以下是使用 Faster R-CNN 实现细胞目标检测的 Python 代码示例:
首先,需要安装以下库:
```python
pip install tensorflow==1.14.0
pip install keras==2.3.1
pip install opencv-python==3.4.3.18
pip install numpy==1.16.4
```
然后,下载 Faster R-CNN 模型文件 `faster_rcnn_inception_v2_coco_2018_01_28.pb` 和标签文件 `mscoco_label_map.pbtxt`。可以从 TensorFlow 官方网站下载这些文件。
接下来,使用以下代码加载模型和标签文件:
```python
import cv2
import numpy as np
import tensorflow as tf
from keras.backend.tensorflow_backend import set_session
config = tf.ConfigProto()
config.gpu_options.per_process_gpu_memory_fraction = 0.5
set_session(tf.Session(config=config))
# Load Faster R-CNN model
model_path = 'faster_rcnn_inception_v2_coco_2018_01_28.pb'
model = cv2.dnn.readNetFromTensorflow(model_path)
# Load label map
label_map_path = 'mscoco_label_map.pbtxt'
label_map = open(label_map_path, 'rt')
label_map_contents = label_map.read().strip()
label_map.close()
# Parse label map
classes = []
class_id = -1
for line in label_map_contents.split('\n'):
if 'id:' in line:
class_id = int(line.split(':')[-1])
elif 'display_name:' in line:
class_name = line.split(':')[-1].strip().strip("'")
classes.append((class_id, class_name))
```
接下来,使用以下代码进行目标检测:
```python
# Load image
image_path = 'cell_image.jpg'
image = cv2.imread(image_path)
# Convert image to blob
blob = cv2.dnn.blobFromImage(image, swapRB=True, crop=False)
# Set input to Faster R-CNN model
model.setInput(blob)
# Run detection
detections = model.forward()
# Display results
for i in range(detections.shape[2]):
confidence = detections[0, 0, i, 2]
if confidence > 0.5:
class_id = int(detections[0, 0, i, 1])
class_name = classes[class_id][1]
box = detections[0, 0, i, 3:7] * np.array([image.shape[1], image.shape[0], image.shape[1], image.shape[0]])
x1, y1, x2, y2 = box.astype('int')
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(image, class_name + ' ' + str(round(confidence * 100, 2)) + '%', (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Display image
cv2.imshow('image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cell_image.jpg` 是需要检测的细胞图片文件。代码会输出检测结果并显示检测后的图片。
相关推荐
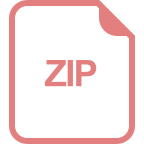
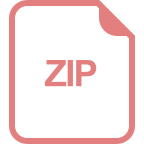










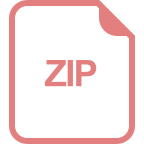
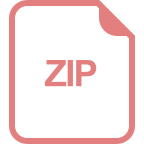