pytesseract.image_to_string如何提高精确度
时间: 2024-06-02 17:13:48 浏览: 58
以下是提高 pytesseract.image_to_string 精确度的一些方法:
1. 调整图像预处理参数:可以通过调整预处理参数来优化图像的清晰度,例如调整图像的对比度、亮度、锐度等。
2. 选择正确的语言:pytesseract支持多种语言,选择正确的语言可以提高识别精度。
3. 增加字典:通过增加字典可以提高识别精度,尤其是针对特定领域的术语和专业词汇。
4. 去除噪声:在图像预处理过程中,可以通过去除噪声等干扰因素来提高识别精度。
5. 增加训练数据:可以通过增加训练数据来优化识别算法,从而提高识别精度。
相关问题
import pytesseract from PIL import Image from pdf2image import convert_from_path pdf_file = 'example.pdf' page = 0 try: # 将PDF文件转换为图像 images = convert_from_path(pdf_file) image = images[page] # 转换为灰度图像 gray_image = image.convert('L') # OCR文本识别,设置参数和预处理操作 text = pytesseract.image_to_string(gray_image, lang='eng', config='--psm 6', noise_filter=True) print(text) except Exception as e: print(f"Error: {e}")优化
可以考虑对图像进行一些预处理,以提高 OCR 文本识别的精确度和速度。以下是一些可能的优化方法:
1. 调整图像大小:将图像调整为合适的大小,可以避免 OCR 识别错误和提高识别速度。
2. 去除噪声:使用图像处理技术去除噪声,可以提高 OCR 识别的精确度和速度。可以尝试使用模糊、锐化等滤波器来去除噪声。
3. 二值化处理:将图像转换为黑白二值图像,可以减少识别错误和提高识别速度。
4. 调整 OCR 参数:根据具体情况调整 OCR 的参数,例如识别语言、识别模式等。
下面是一个优化后的代码示例:
```python
import pytesseract
from PIL import Image
from pdf2image import convert_from_path
pdf_file = 'example.pdf'
page = 0
try:
# 将PDF文件转换为图像
images = convert_from_path(pdf_file, size=(800, None), grayscale=True)
image = images[page]
# 去除噪声和边框
image = image.filter(ImageFilter.MedianFilter())
image = image.filter(ImageFilter.SHARPEN)
image = image.crop((100, 100, image.width - 100, image.height - 100))
# 转换为黑白二值图像
image = image.convert('1')
# OCR文本识别,设置参数和预处理操作
text = pytesseract.image_to_string(image, lang='eng', config='--psm 6')
print(text)
except Exception as e:
print(f"Error: {e}")
```
在这个示例中,我们将图像大小调整为 800 像素宽(高度自适应),然后使用 `MedianFilter` 和 `SHARPEN` 滤波器去除噪声和锐化图像。接着,我们裁剪掉图像边框,转换为黑白二值图像,最后使用 OCR 进行文本识别。这些预处理操作可以根据具体情况进行调整。
在Python应用开发中,如何有效地使用OCR技术来逐页精确识别APP软件窗口中的指定区域文本,并将其识别结果与本地Excel文件中的数据进行精准匹配以检验一致性?
在Python中,要实现这种功能,你需要结合几个关键库和技术:
1. **OCR库**:首先需要一个光学字符识别(OCR)库,如Tesseract(由Google开源)通过`pytesseract`包装器来支持。安装命令通常是`pip install pytesseract opencv-python-headless pillow`。
2. **图像处理**:使用PIL(Pillow)库对屏幕截图进行预处理,比如调整大小、灰度化、二值化等,提高OCR的准确率。
3. **定位区域**:利用类似`pyautogui`这样的屏幕截取工具获取APP窗口的特定区域截图。你需要确定好你想识别的区域的位置和尺寸。
4. **文本提取**:使用OCR技术从预处理后的图片中提取文字,`pytesseract.image_to_string(image)`可以完成这个任务。
5. **Excel操作**:Python的`openpyxl`或`pandas`库可以帮助你读取和操作Excel文件。将识别出的文本与Excel表格的数据进行对比。
示例代码片段可能如下所示:
```python
import pyautogui
import pytesseract
from PIL import Image
import pandas as pd
# 获取屏幕截图并裁剪到指定区域
def capture_region(x, y, w, h):
screenshot = pyautogui.screenshot(region=(x, y, w, h))
img = Image.frombytes('RGB', (w, h), screenshot)
# OCR识别文本
text = pytesseract.image_to_string(img)
# 读取Excel数据
df_excel = pd.read_excel("your_file.xlsx")
# 检查一致性
for excel_row in df_excel.itertuples():
if text == excel_row.some_column_name:
print(f"Match found: {text} with Excel value: {excel_row.some_column_name}")
else:
print(f"Inconsistency: {text} != {excel_row.some_column_name}")
# 使用函数并设置区域坐标
capture_region(x=0, y=0, w=600, h=400) # 这里假设你的目标区域是(0, 0)左上角开始,宽度高度分别为600px和400px
阅读全文
相关推荐
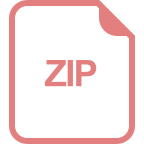
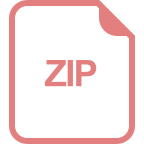
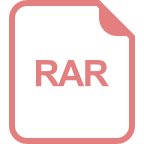
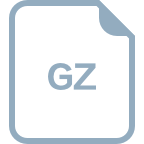
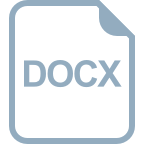
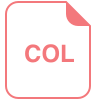
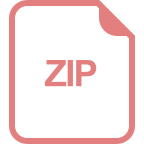
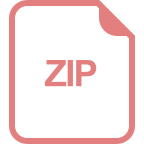
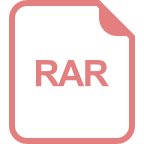
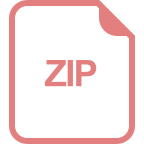
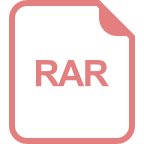
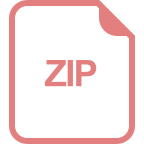
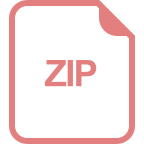