Description 现在有一大批规模为 n 的无序整数,需要请您找出前 m 大的数据。 Input 包含2行。 第一行:两个整数 n 和 m ,分别代表数据规模 n ,需要返回的数据规模 m 。 n 和 m 的取值范围[0,1000000] 第二行: n 个整数,每个数据的取值范围为[0,1000000] Output 规模数据中的前 m 大的数据,从大到小依次输出。 注意:只输出前 m 个数据即可。 Sample Input 10 3 96 36 18 98 42 96 89 78 96 92 Sample Output 98 96 96,用c语言实现代码。
时间: 2024-03-18 13:41:08 浏览: 68
好的,这是一个经典的 Top K 问题。我们可以使用堆排序来解决这个问题。具体来说,我们可以先建立一个大小为 m 的小根堆,然后遍历整个数组,对于每个元素,如果它比堆顶元素大,就把堆顶元素弹出并将这个元素插入堆中。最终,堆中的元素就是前 m 大的元素。时间复杂度为 O(nlogm)。
下面是C语言的代码实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct heap {
int *data;
int size;
int capacity;
} heap;
void swap(int *a, int *b) {
int t = *a;
*a = *b;
*b = t;
}
heap *create(int capacity) {
heap *h = (heap *) malloc(sizeof(heap));
h->data = (int *) malloc(sizeof(int) * (capacity + 1));
h->size = 0;
h->capacity = capacity;
return h;
}
void destroy(heap *h) {
if (h != NULL) {
free(h->data);
free(h);
}
}
void push(heap *h, int value) {
if (h->size < h->capacity) {
h->size++;
h->data[h->size] = value;
int i = h->size;
while (i > 1 && h->data[i] < h->data[i / 2]) {
swap(&h->data[i], &h->data[i / 2]);
i /= 2;
}
} else if (value > h->data[1]) {
h->data[1] = value;
int i = 1;
while (i * 2 <= h->size) {
int j = i * 2;
if (j + 1 <= h->size && h->data[j + 1] < h->data[j]) {
j++;
}
if (h->data[i] > h->data[j]) {
swap(&h->data[i], &h->data[j]);
i = j;
} else {
break;
}
}
}
}
int main() {
int n, m;
scanf("%d%d", &n, &m);
heap *h = create(m);
for (int i = 0; i < n; i++) {
int x;
scanf("%d", &x);
push(h, x);
}
for (int i = h->size; i >= 1; i--) {
printf("%d ", h->data[i]);
}
printf("\n");
destroy(h);
return 0;
}
```
阅读全文
相关推荐
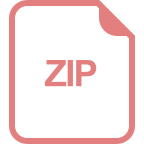
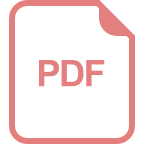















